FastAPI is a speedy and light-weight internet framework for constructing fashionable software programming interfaces utilizing Python 3.6 and above. On this tutorial, we’ll stroll via the fundamentals of constructing an app with FastAPI, and also you’ll get an inkling of why it was nominated as one of many greatest open-source frameworks of 2021.
When you’re able to develop your personal FastAPI apps, you received’t should look far to discover a place to host them. Kinsta’s Software Internet hosting and Database Internet hosting providers present a Platform as a Service that’s robust on Python.
Let’s study the fundamentals first.
Benefits of FastAPI
Beneath are among the benefits the FastAPI framework brings to a undertaking.
- Pace: Because the title implies, FastAPI is a really quick framework. Its velocity is akin to that of Go and Node.js, that are typically thought of to be among the many quickest choices for constructing APIs.
- Straightforward to study and code: FastAPI has already found out virtually every part you will have to make a production-ready API. As a developer utilizing FastAPI, you don’t have to code every part from scratch. With only some traces of code, you may have a RESTful API prepared for deployment.
- Complete documentation: FastAPI makes use of the OpenAPI documentation requirements, so documentation may be dynamically generated. This documentation offers detailed details about FastAPI’s endpoints, responses, parameters, and return codes.
- APIs with fewer bugs: FastAPI helps customized knowledge validation, which permits builders to construct APIs with fewer bugs. FastAPI’s builders boast that the framework leads to fewer human-induced bugs — as a lot as 40% much less.
- Sort hints: The categories module was launched in Python 3.5. This allows you to declare the
sort
of a variable. When the kind of a variable is said, IDEs are capable of present higher help and predict errors extra precisely.
Find out how to Get Began With FastAPI
To observe this tutorial and get began with FastAPI, you’ll have to do just a few issues first.
Guarantee that you’ve a programmer’s textual content editor/IDE, similar to Visible Studio Code. Different choices embody Elegant Textual content and Espresso.
It’s a standard apply to have your Python apps and their situations working in digital environments. Digital environments enable completely different package deal units and configurations to run concurrently, and keep away from conflicts as a result of incompatible package deal variations.
To create a digital surroundings, open your terminal and run this command:
$ python3 -m venv env
You’ll additionally have to activate the digital surroundings. The command to try this will range relying on the working system and shell that you just’re utilizing. Listed below are some CLI activation examples for numerous environments:
# On Unix or MacOS (bash shell):
/path/to/venv/bin/activate
# On Unix or MacOS (csh shell):
/path/to/venv/bin/activate.csh
# On Unix or MacOS (fish shell):
/path/to/venv/bin/activate.fish
# On Home windows (command immediate):
pathtovenvScriptsactivate.bat
# On Home windows (PowerShell):
pathtovenvScriptsActivate.ps1
(Some Python-aware IDEs may also be configured to activate the present digital surroundings.)
Now, set up FastAPI:
$ pip3 set up fastapi
FastAPI is a framework for constructing APIs, however to check your APIs you’ll want an area internet server. Uvicorn is a lightning-fast Asynchronous Server Gateway Interface (ASGI) internet server for Python that’s nice for growth. To put in Uvicorn, run this command:
$ pip3 set up "uvicorn[standard]"
Upon profitable set up, create a file named foremost.py inside your undertaking’s working listing. This file might be your software entry level.
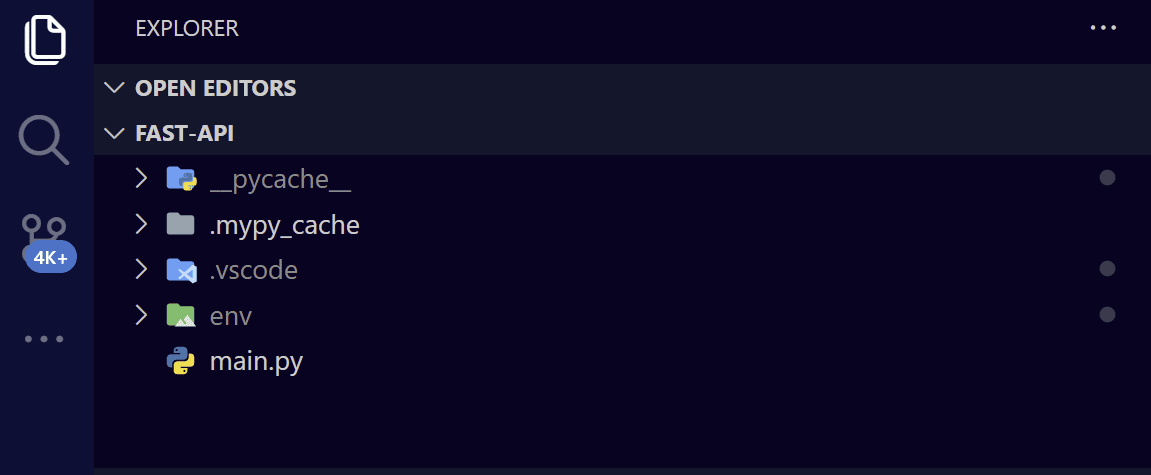
A Fast FastAPI Instance
You’ll take a look at your FastAPI set up by shortly organising an instance endpoint. In your foremost.py file, paste within the following code, then save the file:
# foremost.py
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def root():
return {"greeting":"Good day world"}
The above snippet creates a primary FastAPI endpoint. Beneath is a abstract of what every line does:
from fastapi import FastAPI
: The performance in your API is supplied by the FastAPI Python class.app = FastAPI()
: This creates a FastAPI occasion.@app.get("/")
: This can be a python decorator that specifies to FastAPI that the operate under it’s accountable for request dealing with.@app.get("/")
: This can be a decorator that specifies the route. This creates aGET
technique on the positioning’s route. The result’s then returned by the wrapped operate.- Different doable operations which might be used to speak embody
@app.submit()
,@app.put()
,@app.delete()
,@app.choices()
,@app.head()
,@app.patch()
, and@app.hint()
.
Within the recordsdata listing, run the next command in your terminal to begin the API server:
$ uvicorn foremost:app --reload
On this command, foremost
is the title of your module. The app
object is an occasion of your software, and is imported into the ASGI server. The --reload
flag tells the server to reload robotically once you make any modifications.
It is best to see one thing like this in your terminal:
$ uvicorn foremost:app --reload
INFO: Will look ahead to modifications in these directories: ['D:WEB DEVEunitTestsfast-api']
INFO: Uvicorn working on http://127.0.0.1:8000 (Press CTRL+C to give up)
INFO: Began reloader course of [26888] utilizing WatchFiles
INFO: Began server course of [14956]
INFO: Ready for software startup.
INFO: Software startup full.
In your browser, navigate to http://localhost:8000
to verify that your API is working. It is best to see “Good day”: “World” as a JSON object on the web page. This illustrates how straightforward it’s to create an API with FastAPI. All you needed to do was to outline a route and return your Python dictionary, as seen on line six of the snippet above.
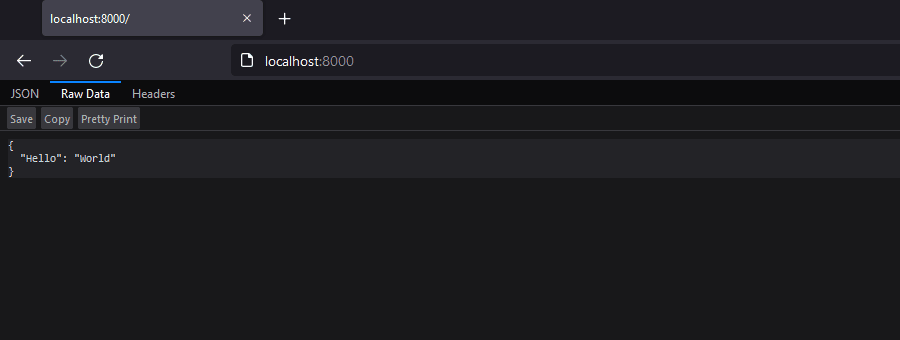
Utilizing Sort Hints
Should you use Python, you’re used to annotating variables with primary knowledge varieties similar to int
, str
, float
, and bool
. Nevertheless, from Python model 3.9, superior knowledge constructions have been launched. This lets you work with knowledge constructions similar to dictionaries
, tuples
, and lists
. With FastAPI’s sort hints, you may construction the schema of your knowledge utilizing pydantic fashions after which, use the pydantic fashions to sort trace and profit from the info validation that’s supplied.
Within the instance under, using sort hints in Python is demonstrated with a easy meal worth calculator, calculate_meal_fee
:
def calculate_meal_fee(beef_price: int, meal_price: int) -> int:
total_price: int = beef_price + meal_price
return total_price
print("Calculated meal payment", calculate_meal_fee(75, 19))
Notice that sort hints don’t change how your code runs.
FastAPI Interactive API Documentation
FastAPI makes use of Swagger UI to supply automated interactive API documentation. To entry it, navigate to http://localhost:8000/docs
and you will notice a display with all of your endpoints, strategies, and schemas.
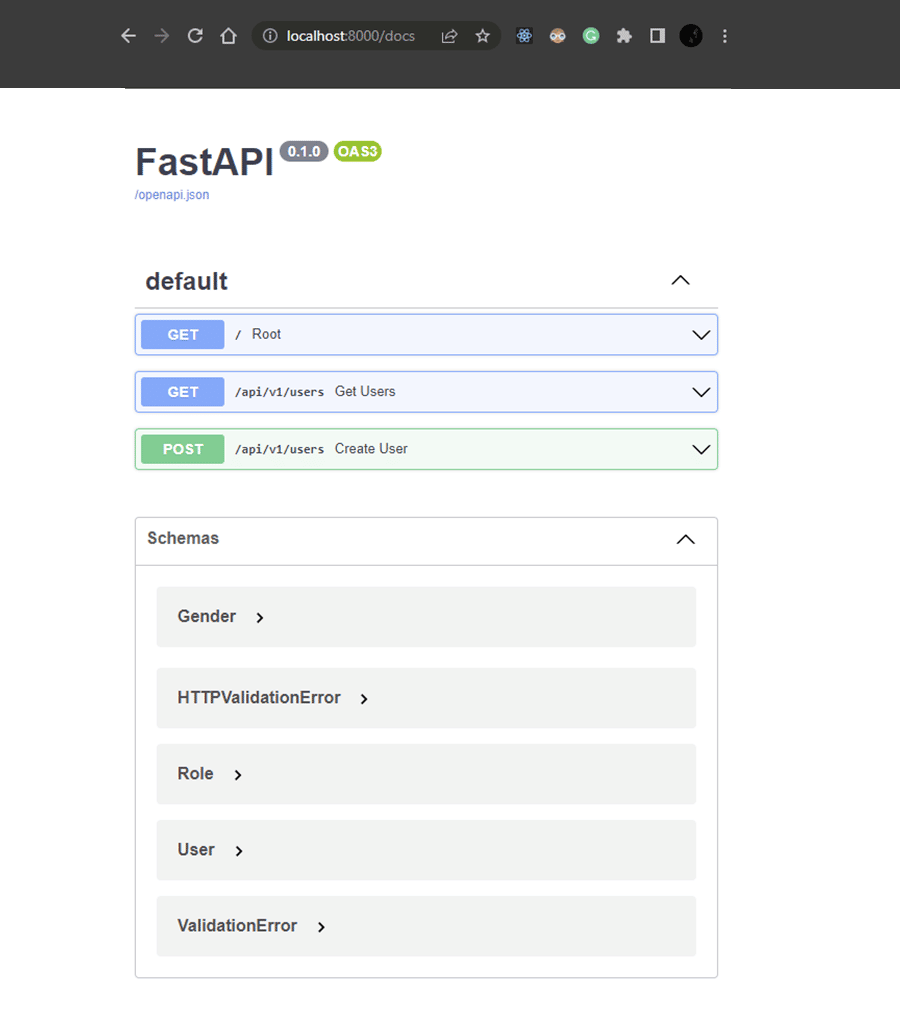
This automated, browser-based API documentation is supplied by FastAPI, and also you don’t have to do the rest to benefit from it.
Another browser-based API documentation, additionally supplied by FastAPI, is Redoc. To entry Redoc, navigate to http://localhost:8000/redoc
, the place you’ll be introduced with an inventory of your endpoints, the strategies, and their respective responses.
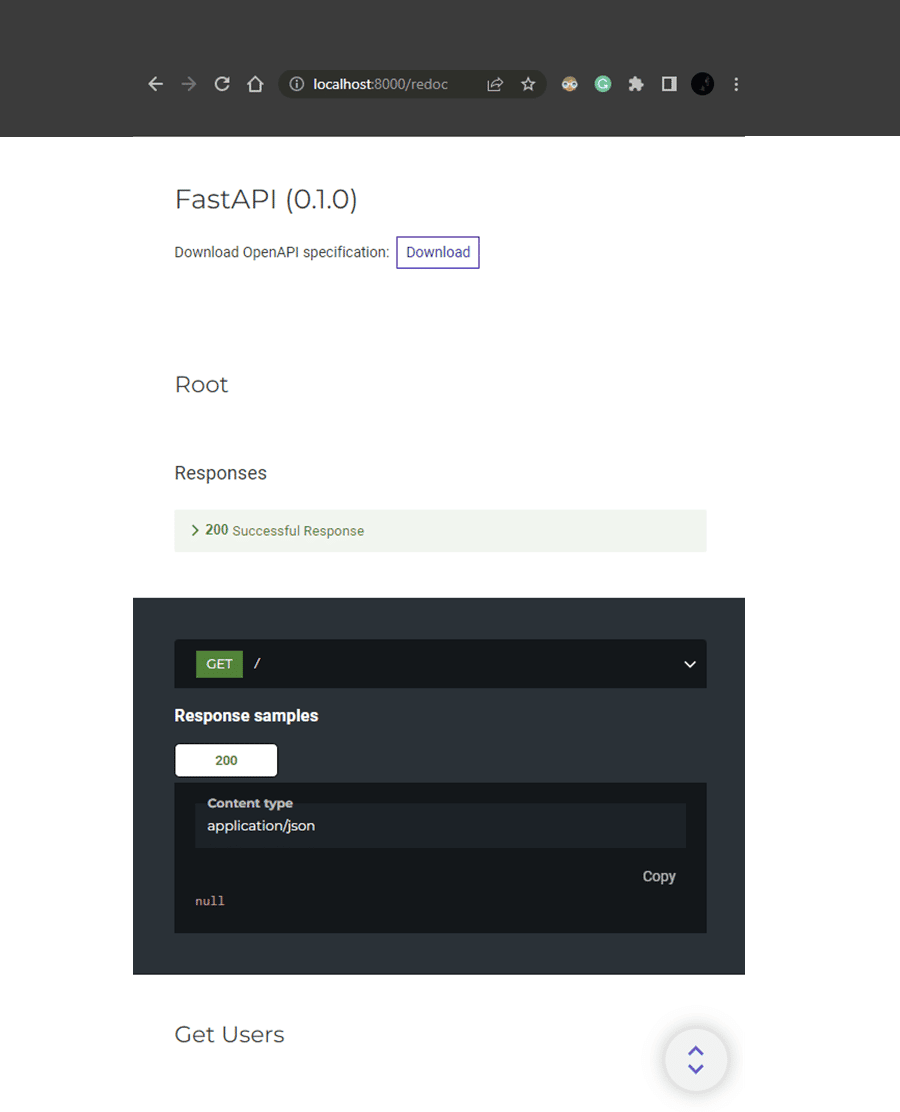
Setting Up Routes in FastAPI
The @app
decorator lets you specify the route’s technique, similar to @app.get
or @app.submit
, and helps GET
, POST
, PUT
, and DELETE
, in addition to the much less frequent choices, HEAD
, PATCH
, and TRACE
.
Constructing Your App With FastAPI
On this tutorial, you’ll be walked via constructing a CRUD software with FastAPI. The applying will be capable of:
- Create a person
- Learn a person’s database file
- Replace an present person
- Delete a specific person
To execute these CRUD operations, you’ll create strategies that expose the API endpoints. The consequence might be an in-memory database that may retailer an inventory of customers.
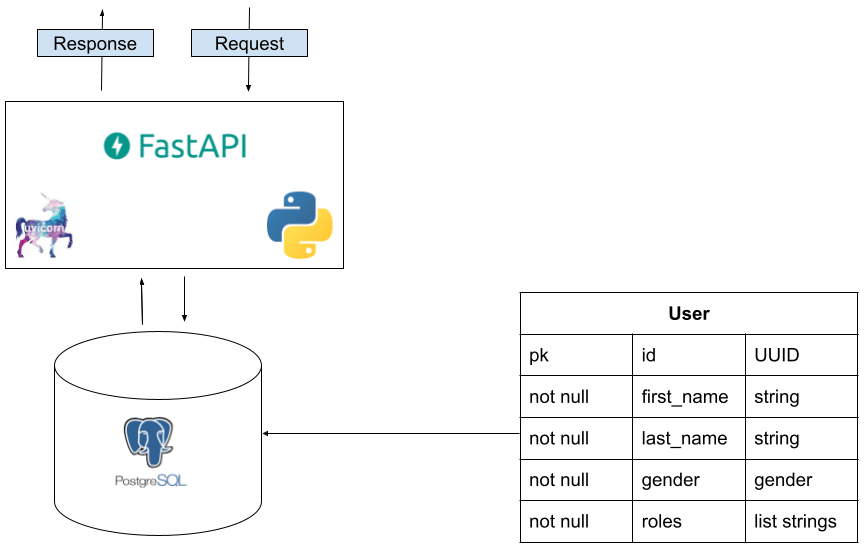
You’ll use the pydantic library to carry out knowledge validation and settings administration utilizing Python sort annotations. For the needs of this tutorial, you’ll declare the form of your knowledge as lessons with attributes.
This tutorial will use the in-memory database. That is to shortly get you began with utilizing FastAPI to construct your APIs. Nevertheless, for manufacturing, you may make use of any database of your selecting, similar to PostgreSQL, MySQL, SQLite, and even Oracle.
Constructing the App
You’ll start by creating your person mannequin. The person mannequin could have the next attributes:
id
: A Common Distinctive Identifier (UUID)first_name
: The primary title of the personlast_name
: The final title of the persongender
: The gender of the personroles
, which is an inventory containingadmin
andperson
roles
Begin by creating a brand new file named fashions.py in your working listing, then paste the next code into fashions.py to create your mannequin:
# fashions.py
from typing import Listing, Non-compulsory
from uuid import UUID, uuid4
from pydantic import BaseModel
from enum import Enum
from pydantic import BaseModel
class Gender(str, Enum):
male = "male"
feminine = "feminine"
class Position(str, Enum):
admin = "admin"
person = "person"
class Person(BaseModel):
id: Non-compulsory[UUID] = uuid4()
first_name: str
last_name: str
gender: Gender
roles: Listing[Role]
Within the code above:
- Your
Person
class extendsBaseModel
, which is then imported frompydantic
. - You outlined the attributes of the person, as mentioned above.
The following step is to create your database. Substitute the contents of your foremost.py file with the next code:
# foremost.py
from typing import Listing
from uuid import uuid4
from fastapi import FastAPI
from fashions import Gender, Position, Person
app = FastAPI()
db: Listing[User] = [
User(
id=uuid4(),
first_name="John",
last_name="Doe",
gender=Gender.male,
roles=[Role.user],
),
Person(
id=uuid4(),
first_name="Jane",
last_name="Doe",
gender=Gender.feminine,
roles=[Role.user],
),
Person(
id=uuid4(),
first_name="James",
last_name="Gabriel",
gender=Gender.male,
roles=[Role.user],
),
Person(
id=uuid4(),
first_name="Eunit",
last_name="Eunit",
gender=Gender.male,
roles=[Role.admin, Role.user],
),
]
In foremost.py:
- You initialized
db
with a sort ofListing
, and handed within thePerson
mannequin - You created an in-memory database with 4 customers, every with the required attributes similar to
first_name
,last_name
,gender
, androles
. The personEunit
is assigned the roles ofadmin
andperson
, whereas the opposite three customers are assigned solely the position ofperson
.
Learn Database Data
You have got efficiently arrange your in-memory database and populated it with customers, so the subsequent step is to arrange an endpoint that may return an inventory of all customers. That is the place FastAPI is available in.
In your foremost.py file, paste the next code slightly below your Good day World
endpoint:
# foremost.py
@app.get("/api/v1/customers")
async def get_users():
return db
This code defines the endpoint /api/v1/customers
, and creates an async operate, get_users
, which returns all of the contents of the database, db
.
Save your file, and you may take a look at your person endpoint. Run the next command in your terminal to begin the API server:
$ uvicorn foremost:app --reload
In your browser, navigate to http://localhost:8000/api/v1/customers
. This could return an inventory of all of your customers, as seen under:
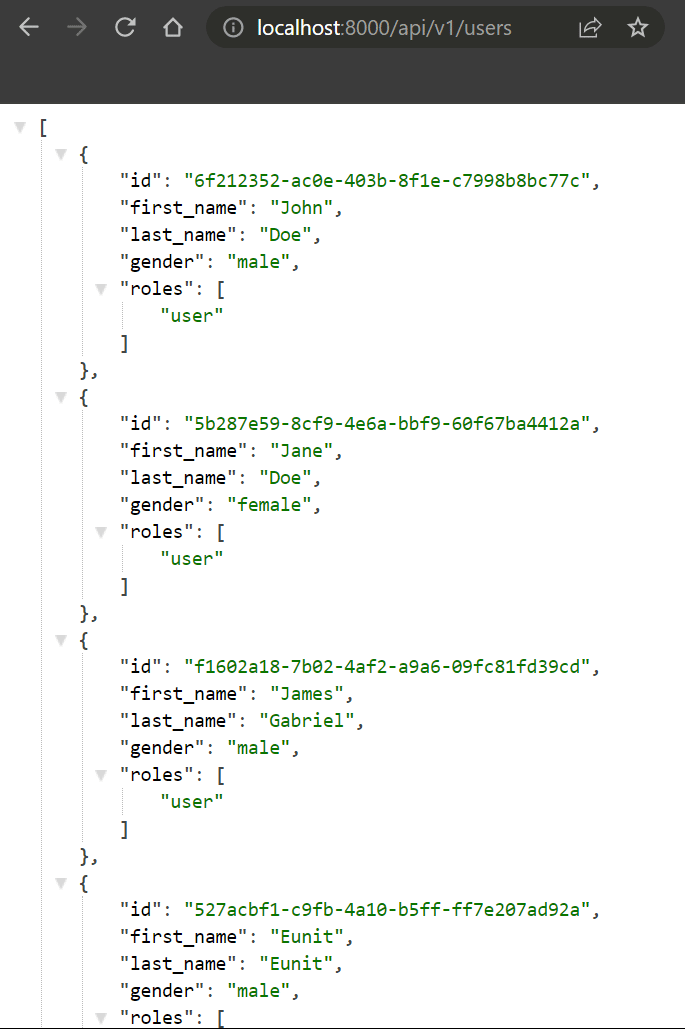
At this stage, your foremost.py file will seem like this:
# foremost.py
from typing import Listing
from uuid import uuid4
from fastapi import FastAPI
from fashions import Gender, Position, Person
app = FastAPI()
db: Listing[User] = [
User(
id=uuid4(),
first_name="John",
last_name="Doe",
gender=Gender.male,
roles=[Role.user],
),
Person(
id=uuid4(),
first_name="Jane",
last_name="Doe",
gender=Gender.feminine,
roles=[Role.user],
),
Person(
id=uuid4(),
first_name="James",
last_name="Gabriel",
gender=Gender.male,
roles=[Role.user],
),
Person(
id=uuid4(),
first_name="Eunit",
last_name="Eunit",
gender=Gender.male,
roles=[Role.admin, Role.user],
),
]
@app.get("/")
async def root():
return {"Good day": "World",}
@app.get("/api/v1/customers")
async def get_users():
return db
Create Database Data
The following step is to create an endpoint to create a brand new person in your database. Paste the next snippet into your foremost.py file:
# foremost.py
@app.submit("/api/v1/customers")
async def create_user(person: Person):
db.append(person)
return {"id": person.id}
On this snippet, you outlined the endpoint to submit a brand new person and made use of the @app.submit
decorator to create a POST
technique.
You additionally created the operate create_user
, which accepts person
of the Person
mannequin, and appended (added) the newly created person
to the database, db
. Lastly, the endpoint returns a JSON object of the newly created person’s id
.
You’ll have to use the automated API documentation supplied by FastAPI to check your endpoint, as seen above. It is because you can not make a submit request utilizing the net browser. Navigate to http://localhost:8000/docs
to check utilizing the documentation supplied by SwaggerUI.
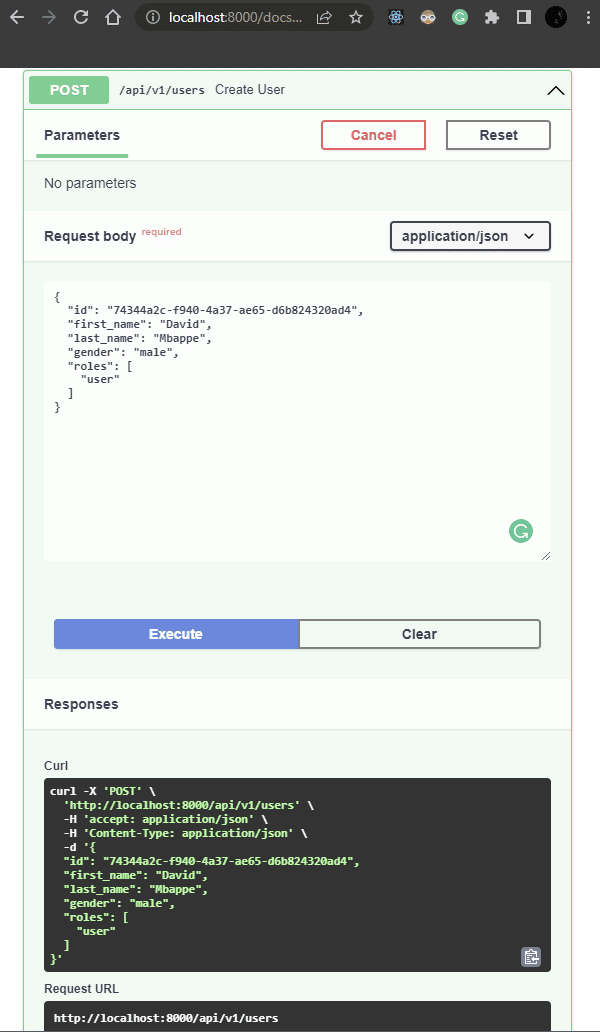
Delete Database Data
Because you’re constructing a CRUD app, your software might want to have the power to delete a specified useful resource. For this tutorial, you’ll create an endpoint to delete a person.
Paste the next code into your foremost.py file:
# foremost.py
from uuid import UUID
from fastapi HTTPException
@app.delete("/api/v1/customers/{id}")
async def delete_user(id: UUID):
for person in db:
if person.id == id:
db.take away(person)
return
increase HTTPException(
status_code=404, element=f"Delete person failed, id {id} not discovered."
)
Right here’s a line-by-line breakdown of how that code works:
@app.delete("/api/v1/customers/{id}")
: You created the delete endpoint utilizing the@app.delete()
decorator. The trail continues to be/api/v1/customers/{id}
, however then it retrieves theid
, which is a path variable equivalent to the id of the person.async def delete_user(id: UUID):
: Creates thedelete_user
operate, which retrieves theid
from the URL.for person in db:
: This tells the app to loop via the customers within the database, and test if theid
handed matches with a person within the database.db.take away(person)
: If theid
matches a person, the person might be deleted; in any other case, anHTTPException
with a standing code of 404 might be raised.
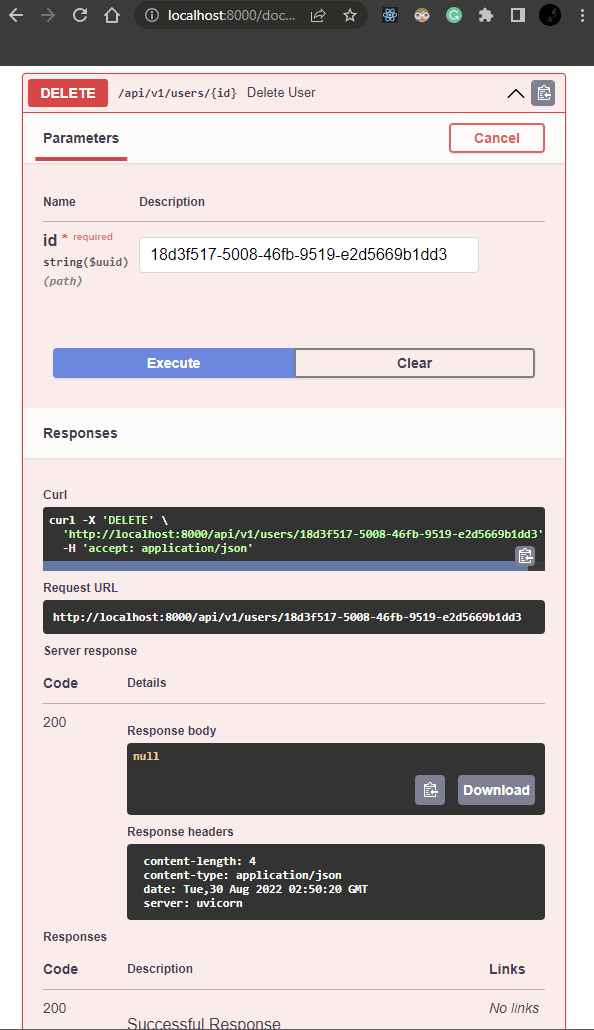
Replace Database Data
You will create an endpoint to replace a person’s particulars. The small print that may be up to date embody the next parameters: first_name
, last_name
, and roles
.
In your fashions.py file, paste the next code underneath your Person
mannequin, that’s after the Person(BaseModel):
class:
# fashions.py
class UpdateUser(BaseModel):
first_name: Non-compulsory[str]
last_name: Non-compulsory[str]
roles: Non-compulsory[List[Role]]
On this snippet, the category UpdateUser
extends BaseModel
. You then set the updatable person parameters, similar to first_name
, last_name
, and roles
, to be elective.
Now you’ll create an endpoint to replace a specific person’s particulars. In your foremost.py file, paste the next code after @app.delete
decorator:
# foremost.py
@app.put("/api/v1/customers/{id}")
async def update_user(user_update: UpdateUser, id: UUID):
for person in db:
if person.id == id:
if user_update.first_name just isn't None:
person.first_name = user_update.first_name
if user_update.last_name just isn't None:
person.last_name = user_update.last_name
if user_update.roles just isn't None:
person.roles = user_update.roles
return person.id
increase HTTPException(status_code=404, element=f"Couldn't discover person with id: {id}")
Within the code above, you’ve performed the next:
- Created
@app.put("/api/v1/customers/{id}")
, the replace endpoint. It has a variable parameterid
that corresponds to the id of the person. - Created a way referred to as
update_user
, which takes within theUpdateUser
class andid
. - Used a
for
loop to test if the person related to the handedid
is within the database. - Checked if any of the person’s parameters are
just isn't None
(not null). If any parameter, similar tofirst_name
,last_name
, orroles
, just isn’t null, then it’s up to date. - If the operation is profitable, the person id is returned.
- If the person wasn’t situated, an
HTTPException
exception with a standing code of 404 and a message ofCouldn't discover person with id: {id}
is raised.
To check this endpoint, make sure that your Uvicorn server is working. If it’s not working, enter this command:
uvicorn foremost:app --reload
Beneath is a screenshot of the take a look at.
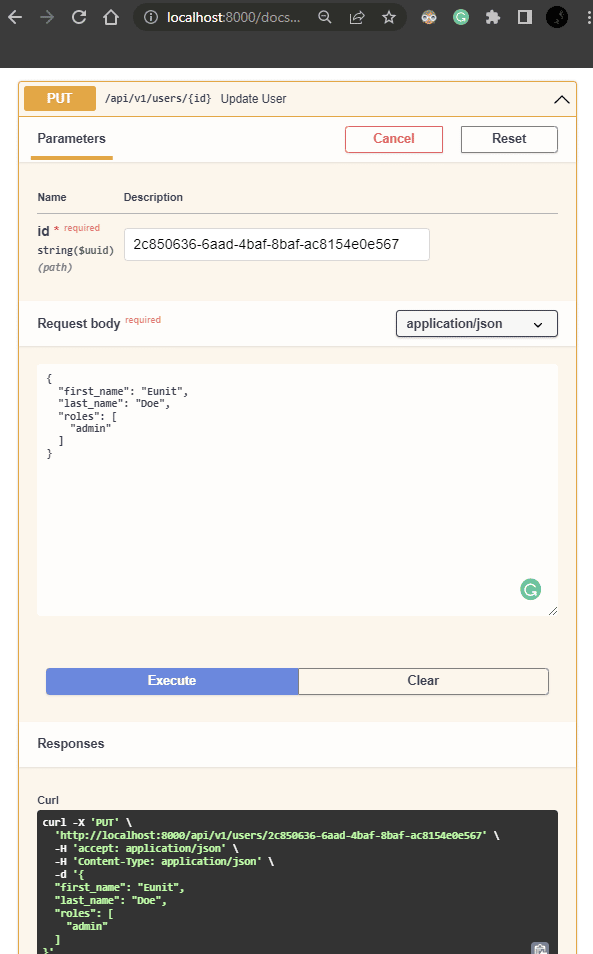
Abstract
On this tutorial, you’ve discovered concerning the FastAPI framework for Python and noticed for your self how shortly you may get a FastAPI-powered software up and working. You discovered tips on how to construct CRUD API endpoints utilizing the framework — creating, studying, updating, and deleting database data.
Now, if you wish to take your internet app growth to the subsequent stage, remember to try Kinsta’s platform for Software Internet hosting and Database Internet hosting. Like FastAPI, it’s powerfully easy.
Save time, prices and maximize website efficiency with:
- Immediate assist from WordPress internet hosting consultants, 24/7.
- Cloudflare Enterprise integration.
- World viewers attain with 35 knowledge facilities worldwide.
- Optimization with our built-in Software Efficiency Monitoring.
All of that and far more, in a single plan with no long-term contracts, assisted migrations, and a 30-day-money-back-guarantee. Take a look at our plans or speak to gross sales to seek out the plan that’s best for you.