Vite has grow to be the lynchpin of frontend toolchains. This construct device additionally has been adopted by SvelteKit, which is the official utility framework for Svelte. So, I feel it’s honest to say that Vite has grow to be the primary selection dev device for Svelte.
Vitest is a comparatively new, Vite-native unit testing framework. It holds the promise of being Vite’s best testing companion, however does it ship?
On this article, I’ll discover the next:
Testing frameworks for Svelte and Vite
Presently, Svelte doesn’t advocate a specific unit testing framework, and it doesn’t advocate for a specific testing technique. The official web site gives some primary recommendation. In principle, you should use any JavaScript unit testing library you want for testing, and your frontend toolchain will finally spit out a vanilla JavaScript bundle, proper?
Effectively, that is the crux of the issue; you might be coping with completely different permutations of syntaxes and codecs associated to JavaScript. These codecs are remodeled into one other format by your toolchain. It may be tough to combine instruments right into a toolchain when they don’t seem to be talking the identical syntax, and should not cooperating nicely.
Vite gives a quick dev setting by utilizing native ECMAScript Modules (ESM) to offer on-demand file serving. It performs on-the-fly atomic translation of Svelte to JavaScript. Many unit testing frameworks have been constructed utilizing CommonJS modules, which is an alternate module commonplace. Utilizing Vite to handle the interpretation of the recordsdata after which passing them onto a testing framework constructed with a special commonplace can create friction.
Vite’s Unit Testing story hasn’t been clear although. Current choices like Jest have been created in a special context. There may be a whole lot of duplication between Jest and Vite, forcing customers to configure two completely different pipelines.
Jest might be the most well-liked unit testing framework; it got here out on high within the State of JS Survey in 2021. Jest’s integration with Vite is patchy. There’s a library referred to as vite-jest, which goals to offer first-class Vite integration for Jest; nevertheless, it’s at the moment a work-in-progress.
Vite-jest doesn’t point out Svelte, and should not work with Svelte. For SvelteKit, there’s an experimental library referred to as svelte-add-jest. The underside line is that there’s not a transparent, dependable approach of utilizing Jest with Vite and Svelte.
In any case, as talked about by the Vitest crew, there’s a duplication of effort between Jest and Vite. You find yourself with a pipeline for improvement and a pipeline for testing. For Jest, chances are you’ll be utilizing Babel with a plugin to translate from Svelte to JavaScript. Then, you want to sew some accompanying items collectively to make it work. It turns into a little bit of a Rube Goldberg Machine.
I wrote about this in one other article, Testing a Svelte app with Jest. I created a starter template to make use of Vite, Svelte, Jest, and Svelte Testing Library collectively. It required roughly 10 dependencies, and two extra config recordsdata (.babelrc
and jest.config.json
) to create the testing pipeline.
Having this type of setup is fragile, particularly once you don’t totally perceive the internal workings of the instruments. I might be praying {that a} change to a type of dependencies doesn’t break the chain! Extra hyperlinks, extra risk for failure! Not a very good feeling for those who grow to be the maintainer of a mission!
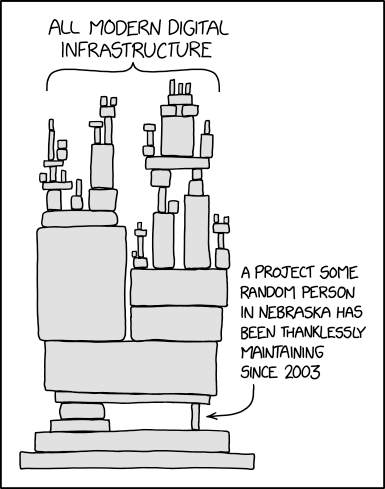
In abstract, a extra built-in answer is preferable. A Vite-native answer is even higher. A Vite-native answer with a Jest-compatible API could be higher nonetheless! Doing all of it in a single configuration file could be Nirvana! That is probably what Vitest can ship.
First, let’s skim over the API to see how our assessments will look.
Writing assessments with Vitest
You’ll be able to learn the Vitest API documentation for extra detailed data, however to overview the important thing factors I’ll present a fast API overview.
By default, Vitest appears to be like for filenames that finish with both .spec.js
or .check.js
. You’ll be able to configure it in another way for those who desire. I identify my check recordsdata <component-name>.spec.js
and place them alongside my part recordsdata.
The API is appropriate with Chai assertions and Jest count on. When you’ve got used these earlier than, this will probably be acquainted. The important thing bits are:
describe
blocks: used to group associated assessments right into a check suite; a set permits you to arrange your assessments so experiences are clear; you may nest them too for those who want to do additional aggregationcheck
orit
blocks: used to create a person checkcount on
statements: once you’re writing assessments, you want to verify that values meet sure circumstances – these are known as assertions; thecount on
perform gives entry to a number of “matcher” features that allow you to validate various kinds of issues (e.g.,toBeNull
,toBeTruthy
)
Here’s a primary skeleton of how a check suite with one check for our Todo
part would possibly look:
import {describe, count on, it} from 'vitest'; import Todo from "./Todo.svelte"; describe("Todo", () => { let occasion = null; beforeEach(() => { //create occasion of the part and mount it }) afterEach(() => { //destory/unmount occasion }) check("that the Todo is rendered", () => { count on(occasion).toBeDefined(); }) })
When you may use Vitest by itself to run assessments, you’d need to create an occasion of the part after which mount it to a doc. We will do that within the beforeEach
perform. You’d additionally most likely must destroy or unmount this occasion in afterEach
. That is cumbersome; it’s the sort of boilerplate that’s finest prevented.
It’s extra frequent to make use of the Svelte Testing Library which helps push you in direction of good testing practices. It has a render
perform that takes care of the rendering of the part for us and does it in-memory utilizing jsdom. You can too get extra handy matcher features, similar to toBeInTheDocument()
by jest-dom library.
First, you’ll set up the libraries utilizing this command:
npm i -D @testing-library/svelte jest-dom jsdom
I’m not totally certain if you want to set up jsdom your self. I could have been prompted to put in it; I don’t recall precisely!
Now, we are able to deal with writing assessments. You’ll be able to see that we go our part identify and props to the render
perform to render our part. Then, we’ve entry to HTML output by means of an implicit display
variable. We will run question strategies to search out the web page parts that we’d like to check.
import { render, display } from "@testing-library/svelte"; import Todo from "./Todo.svelte"; describe("Todo", () => { const todoDone = { id: 1, textual content: "purchase milk", finished: true }; const todoNotDone = { id: 2, textual content: "do laundry", finished: false }; check("reveals the todo textual content when rendered", () => { render(Todo, { props: { todo: todoDone } }); count on(display.getByLabelText("Accomplished")).toBeInTheDocument(); // checkbox count on(display.getByText(todoDone.textual content)).toBeInTheDocument(); }); });
Migrating a mission from Jest to Vitest
There’s a brief migration information on the Vitest web site.
(deep breath)
Let’s go!
I’ll fork a Todo app that I beforehand made after which examined with Jest and the Svelte Testing Library. I take advantage of the <component_name>.spec.js
naming conference for my assessments alongside the accompanying part. It has 98.07% protection.
The Todo app has the next options:
- Listing todos: When no gadgets stay on the checklist, the app shows the message, “Congratulations, all finished!”
- File progress: Customers can mark todos accomplished, and unmark todos that also want consideration; accomplished todos are styled in another way with grey textual content and a strikethrough ornament.
- Add new todos: Customers could add a brand new todo, however the app prohibits the addition of an empty todo
Right here’s an outline of the parts:
Set up
First, we set up Vitest. I put in it with npm, however you should use your bundle supervisor of selection:
# with npm npm i -D vitest # or with yarn yarn add -D vitest # or with pnpm pnpm add -D vitest
Subsequent, let’s verify that we’ve the newest variations of all the things. Vitest requires Vite v2.7.10+ and Node v14+.
For Vite, I used to be utilizing v2.6.4, so I must replace that. The quickest approach is to run: npm i -D vite
.
I used to be utilizing Node is v14.18.1, so no replace was required there. If you want to replace Node, you may comply with this information.
Configuration
In line with the Vitest migration information:
Jest has their globals API enabled by default. Vitest doesn’t. You’ll be able to both allow globals through the
globals
configuration setting or replace your code to make use of imports from thevitest
module as an alternative.
Let’s allow the globals
possibility. Right here’s what our vite.config.js
file appears to be like like with the globals possibility enabled:
import { defineConfig } from "vite"; import { svelte } from "@sveltejs/vite-plugin-svelte"; // https://vitejs.dev/config/ export default defineConfig({ plugins: [svelte()], check: { globals: true, }, });
Now, let’s attempt to run Vitest; the command: npx vitest run
will runs the assessments as soon as.
After working this command, all 16 assessments fail!
There are two kinds of errors:
- Can not discover
@testing-library/svelte
Node modules: That is the Svelte Testing Library that we’re utilizing; we are able to come again to this one ReferenceError
: doc will not be outlined: Thedoc
object is outlined in jsdom; that is used to emulate the DOM API
I assume that the setting is ready to Node by default, the identical transfer Jest made just lately. Let’s change the setting variable to jsdom
:
export default defineConfig({ plugins: [svelte()], check: { globals: true, setting: "jsdom", }, });
And that solves the doc errors. Now, 4 assessments go. However, we nonetheless have seven fails.
The remaining errors are completely different; they’re categorised as an invalid Chai propertyError
. For instance, invalid Chai property: toBeInTheDocument
.
The toBeInTheDocument
and toBeEnabled
features come from the jest-dom library, which is a companion to the Svelte Testing Library. We didn’t want import statements in our check recordsdata beforehand as a result of we configured Jest to incorporate jest-dom. Right here’s the choice from our Jest config file (jest.config.json
):
"setupFilesAfterEnv": ["@testing-library/jest-dom/extend-expect"]
To do the equal in Vitest, we are able to configure a setup file that’s run earlier than every check file. We will set this by means of the setupFiles
possibility.
We’ll create an src/setuptest.js
file that features the next import
assertion:
import "@testing-library/jest-dom";
We will replace our check
object within the vite.config.js
file in order that it appears to be like like this:
export default defineConfig({ plugins: [svelte()], check: { globals: true, setting: "jsdom", setupFiles: ["src/setupTest.js"], }, });
Now, 11 assessments go, and just one fails! We’re nearly there!
FAIL src/parts/Todo.spec.js [ src/components/Todo.spec.js ] Error: Error: Can not discover module @testing-library/svelte/node_modules/@testing-library/dom imported from file:///residence/rob/programming/workspace/js/svelte/svelte-todo-with-tests-(vitest), file:///residence/rob/programming/workspace/js/svelte/svelte-todo-with-tests-(vitest)/node_modules ❯ MessagePort.[nodejs.internal.kHybridDispatch] inner/event_target.js:399:24
The ultimate error has to do with the second import
assertion in to the Todo.spec.js
file:
import { render, display } from "@testing-library/svelte"; import { fireEvent } from "@testing-library/svelte/node_modules/@testing-library/dom";
I’m undecided why I had a second import
assertion like that! The fireEvent
is a part of the identical library as the primary assertion, so we should always be capable of condense each statements right into a single import
like this:
import { render, display, fireEvent } from "@testing-library/svelte";
Are we good?
Sure! All 16 assessments go! 🙌
Now, let’s tidy up our scripts
within the bundle.json
file:
scripts: { "check": "npx vitest", "protection": "npx vitest run --coverage" }
Protection reporting requires an extra bundle, c8. Let’s set up that bundle and run the protection:
npm i -D c8 npm run protection
Right here’s the output:
We’ve been upgraded to 100% protection now (it was 98.07% in Jest). Bonus factors! 🎁
And eventually, we are able to delete the Jest-related dependencies and configurations. Listed below are the instructions I used to scrub up:
rm jest.config.json .babelrc npm uninstall -D @babel/preset-env babel-jest jest jest-transform-stub svelte-jester
We drop two configuration recordsdata and 5 dependencies. I really feel like I’m floating! 🎈😄
Right here’s the GitHub repo for this mission.
Ideas on the Vitest migration expertise
I might give the Vitest migration expertise a seven out of 10. The migration information leaves you quick for those who’re utilizing jsdom, Svelte Testing Library, or jest-dom. It’s a few further, quick steps, which can have you ever scratching your head. If that doc is improved, I might give it a 9 out of 10. It was a pleasing shock to have the ability to get all the things to work with out modifying the check recordsdata.
Efficiency of Vitest’s options
Up to now, we’ve confirmed that a number of of Vitest’s options work:
- Part testing for Svelte
- Use of the Svelte Testing Library with Vitest
- Utilizing jsdom for testing in opposition to an in-memory doc
- Chai built-in for assertions and Jest count on appropriate APIs
- Native code protection through c8
Now, let’s use our Todo mission to have a look at a few of Vitest’s different essential options. I’m attempting to gauge if Vitest is production-ready. Presently, Vitest is at model 0.14.1, so I assume it’s nonetheless thought-about beta.
Good and prompt watch mode
Identical to how Vite works within the browser, Vitest additionally is aware of the graph of your modules. This permits Vitest to do sensible detection and solely rerun assessments associated to modifications. In line with the Vitest crew, it “…feels nearly like HMR, however for assessments”.
Let’s run Vitest in watch mode with npm run check
and alter one of many part recordsdata.
Let’s open the AddTodo.svelte
file and make a breaking change. I’ll take away the disabled
attribute from the button
, which ought to set off a failing check.
Vitest solely reruns the associated check suites (AddTodo
and App
), and we get one failing check case as anticipated! The working of the check suites takes 446ms, whereas to run all check suites takes a minimum of a couple of seconds! This enchancment is nice for productiveness.
Concurrent assessments
We will add .concurrent
to a set or to particular person assessments to run them in parallel:
import { render, display, fireEvent } from "@testing-library/svelte"; import App from "./App.svelte"; describe.concurrent("App", () => { /* all assessments run in parallel */ })
Let’s see if this hastens the assessments! In principle, my app ought to be capable of run all assessments concurrently.
As a baseline, working my 4 check suites took 5.11s from a chilly begin. Working it with the check suites modified to be concurrent took 3.97s. It shaved off over a second! 🎉
Inbuilt TypeScript assist
Testing a TypeScript-Svelte app seems to be working nicely. Johnny Magrippis has a radical video tutorial on this matter. He makes a small foreign money dashboard with SvelteKit and assessments it with Vitest. The code may be present in this GitHub repo.
Take a look at filtering: Focusing on assessments on the command line
Take a look at recordsdata to be focused on the command line may be filtered by passing a reputation/sample as an argument. For instance, the next command will solely run recordsdata that comprise the phrase Listing
:
npx vitest Listing
Within the case of our instance, solely the TodoList.spec
file is run.
Skipping suites and assessments
You can too add .skip
to the describe
or check
features to keep away from working sure suites or assessments.
Right here I skip the Todo
check suite by including .skip
to the describe
perform. As you may see above, the output informs you which of them check suites and assessments are skipped. And, the colour encoding makes them simple to identify.
Utilizing Vitest with SvelteKit
To make use of Vitest with SvelteKit, you’ll want so as to add the testing dependencies:
npm i -D vitest @testing-library/svelte jest-dom jsdom
Subsequent, we have to add the identical configuration that I shared earlier within the Jest to Vitest migration instance. However, the place precisely can we put the configuration?
The short and soiled approach
The strategy I took was to place my configuration in a file referred to as vitest.config.js
within the mission root. This file contained the next code:
import { defineConfig } from "vite"; import { svelte } from "@sveltejs/vite-plugin-svelte"; // https://vitejs.dev/config/ export default defineConfig({ plugins: [svelte({ hot: !process.env.VITEST })], check: { globals: true, setting: "jsdom", setupFiles: ["src/setupTest.js"], }, });
I additionally added the src/setupTest.js
file that imports jest-dom
. This protects us from having so as to add the import
assertion to every file. The src/setupTest.js
file has the next contents:
import "@testing-library/jest-dom";
This works, however am I doing one thing suspect?
I’m undecided. However, there may be one other approach that I’ve seen talked about.
The right approach?
The SvelteKit configuration lives within the svelte.config.js
file positioned within the mission root. There’s a Vite possibility that takes a Vite config object as its worth. It might be good so as to add the Vitest-related choices right here, after which we’d have all the things in a single config. I attempted this, and it didn’t work!
I seen that Johnny Magrippis added a library referred to as vitest-svelte-kit so as to add the Vitest-related choices straight into the svelte.config.js
file. Nonetheless, this doesn’t work routinely. To make use of this method, you want to do the next:
First, set up vitest-svelte-kit:
npm i -D vitest-svelte-kit
Subsequent, add the test-related stuff to your svelte.config.js
file within the vite
object:
import adapter from "@sveltejs/adapter-auto" import preprocess from "svelte-preprocess" /** @kind {import('@sveltejs/package').Config} */ const config = { // Seek the advice of https://github.com/sveltejs/svelte-preprocess // for extra details about preprocessors preprocess: preprocess(), package: { adapter: adapter(), vite: { check: { setting: "jsdom", globals: true, setupFiles: 'src/setupTests.ts', }, }, }, } export default config
Then, create a vitest.config.js
file and expose a perform from the library:
import { extractFromSvelteConfig } from "vitest-svelte-kit" export default extractFromSvelteConfig()
I assume vitest-svelte package hasn’t completely labored out all of the kinks but, nevertheless it labored tremendous for me so far as I went with it.
Later, I hope that there will probably be an adder. Adders are a easy approach so as to add integrations to a SvelteKit mission. An adder would make it potential to incorporate Vitest once you create a brand new app on the command line. That would offer a confirmed path. So, we’re not all the way in which there but with a single config file.
I used to be stunned to see how nicely supported Vitest is already for testing within the browser and in your IDE. Now, let’s check out how Vitest integrates with a Internet UI and an IDE.
Internet UI integration
You should utilize Vitest in an online UI. It requires an extra bundle, and it’s best to run it with the --ui
flag:
npm i -D @vitest/ui
Subsequent, you can begin Vitest by passing the --ui
flag:
npx vitest --ui
Then, you may go to the Vitest UI at http://localhost:51204/__vitest__/
.
Nonetheless, I didn’t see the leads to any browser on Ubuntu! 🙈 I solely noticed a skinny inexperienced line!
IDE integration
You can too use Vitest in an IDE. There’s an extension for VS Code and a plugin for JetBrains merchandise.
I took the VS Code extension for a spin, and it labored nicely. It gives a sidebar view the place you may run assessments. It will possibly kick off a debugging session by bringing you to the code of your failed assessments.
Conclusion
I’m impressed with Vitest. It’s quick, the sensible watch mode is nice, it’s simpler to configure than the competitors, and it has melded the very best practices from different frameworks to offer a well-known testing expertise.
Having the ability to migrate an current mission from Jest to Vitest, with out having to alter the check recordsdata, is a big win. I feel utilizing Vitest with Svelte and SvelteKit is sort of a no brainer.
Whereas I did take Vitest by means of it paces for a small app, I can not say if there are any points when engaged on a bigger mission, or communicate to the way it manages extra complicated check circumstances. I assume you’d be in a pioneering house for those who used it on a manufacturing app; so there’s some aspect of threat there.
In case you’re utilizing Jest in your mission already, you may pilot Vitest alongside Jest while not having to meddle along with your check recordsdata. In case you’re on this camp, this technique would allow you to mitigate the danger.
General, I might advocate utilizing Vitest with Svelte. It has monetary backing, full-time crew members, and a sturdy neighborhood I count on a vibrant future for Vitest!