There isn’t any scarcity of JavaScript libraries and frameworks for contemporary internet builders. One of the ubiquitous libraries is React, which Fb (now Meta) created to assist construct feature-rich functions. React functions historically run in internet browsers, however the Subsequent.js framework extends React performance to the server aspect by way of the JavaScript runtime atmosphere Node.js.
On this article, we’ll have a look at Subsequent.js and React to be able to determine in the event that they’re proper to your subsequent undertaking.
Subsequent.js and React: JavaScript on the Subsequent Stage
A 2022 SlashData survey discovered that there are greater than 17 million JavaScript programmers world wide, main a pack that features well-liked languages like Python and Java. JavaScript can be utilized on each the shopper and server sides, and this versatility means builders can construct full-blown functions utilizing one programming language.
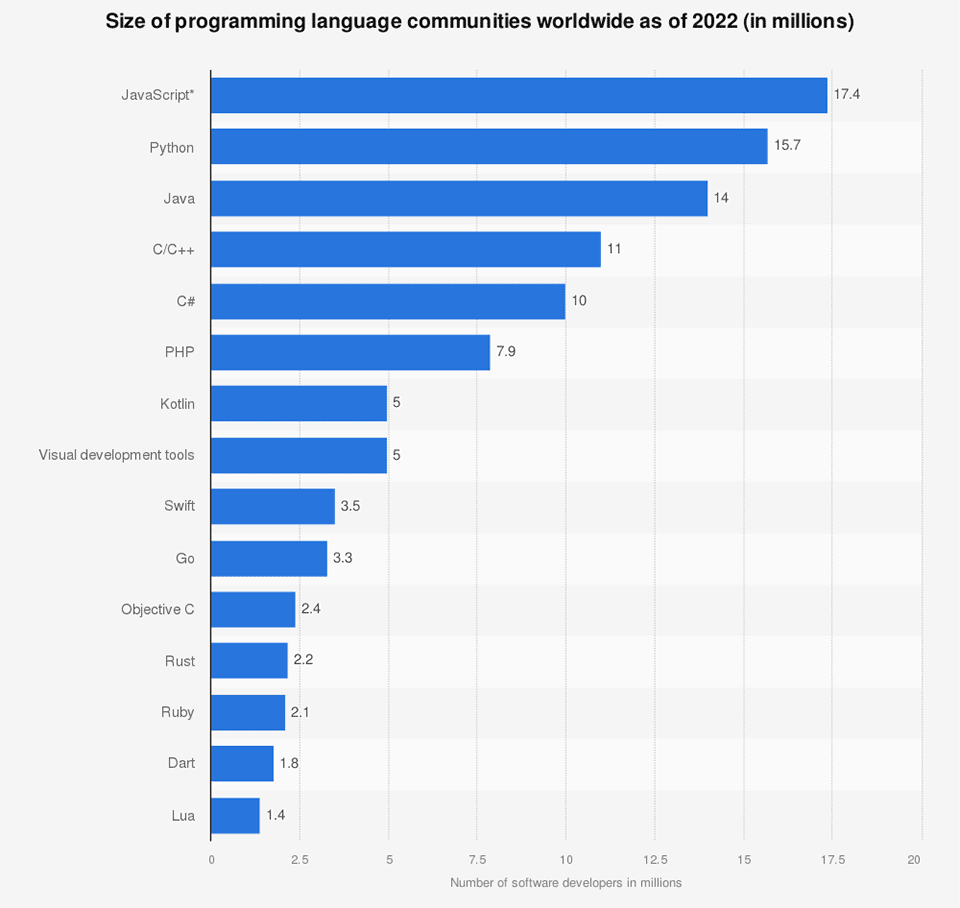
The introduction of JavaScript libraries like React and frameworks like Subsequent.js additional enhanced that improvement. These libraries and frameworks present options that simplify frontend and backend integration. Moreover, builders can lengthen JavaScript capabilities utilizing bundle managers like npm (the Node.js bundle supervisor) to put in JavaScript libraries and instruments. These assets present subtle options that scale back the quantity of code you must write your self.
The extensibility of JavaScript implies that a complete information of its commonest instruments is essential to your success as an online developer.
What Is Subsequent.js?
Initially launched in 2016 by Vercel, Subsequent.js is an open-source React framework that gives the constructing blocks to create high-performance internet functions. Main firms have since adopted it, together with Twitch, TikTok, and Uber, to call a number of.
Subsequent.js gives the most effective developer experiences for constructing quick, Search engine optimization-friendly functions. Beneath are some options of Subsequent.js that make it an distinctive manufacturing framework:
- Hybrid rendering capabilities
- Computerized code-splitting
- Picture optimization
- Constructed-in help for CSS preprocessors and CSS-in-JS libraries
- Constructed-in routing
These options assist Subsequent.js builders save appreciable time on configuration and tooling. You’ll be able to leap straight to constructing your utility, which could help initiatives like the next:
- Ecommerce shops
- Blogs
- Dashboards
- Single-page functions
- Work together consumer interfaces
- Static web sites
What Is React?
React is a JavaScript library used to construct dynamic consumer interfaces. Along with creating internet interfaces, you may construct cellular functions utilizing React Native.
Some advantages of utilizing React embody:
- Improved efficiency: As a substitute of updating every part within the DOM, React makes use of a digital DOM to replace solely the modified parts.
- Closely component-based: When you create a part, you may reuse it repeatedly.
- Straightforward debugging: React functions use a unidirectional information movement – from guardian to youngster parts solely.
Subsequent.js vs React
Though builders usually use Subsequent.js and React for a similar goal, there are some basic variations between the 2.
Ease of Use
It’s straightforward to begin with Subsequent.js and React. Every requires operating single instructions in your terminal utilizing npx
, which is a part of the npm for Node.js.
For Subsequent.js, the best command is:
npx create-next-app
With no further arguments for create-next-app
, the set up will proceed in interactive mode. You can be requested for a undertaking title (which shall be used for the undertaking listing), and whether or not you wish to embody help for TypeScript and the code linter ESLint.
It would look one thing like this:
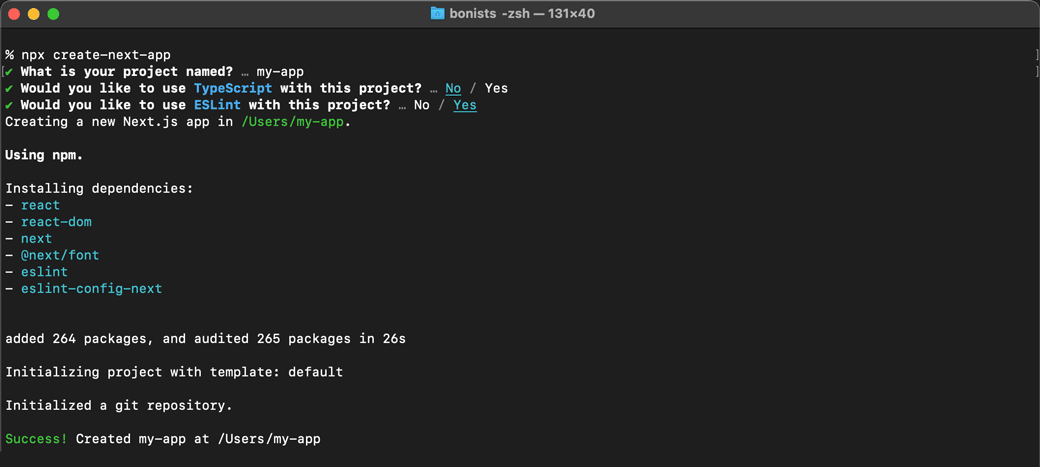
When initializing a React occasion, the best command features a title for the undertaking’s listing:
npx create-react-app new-app
This generates a folder containing all the mandatory preliminary configurations and dependencies:
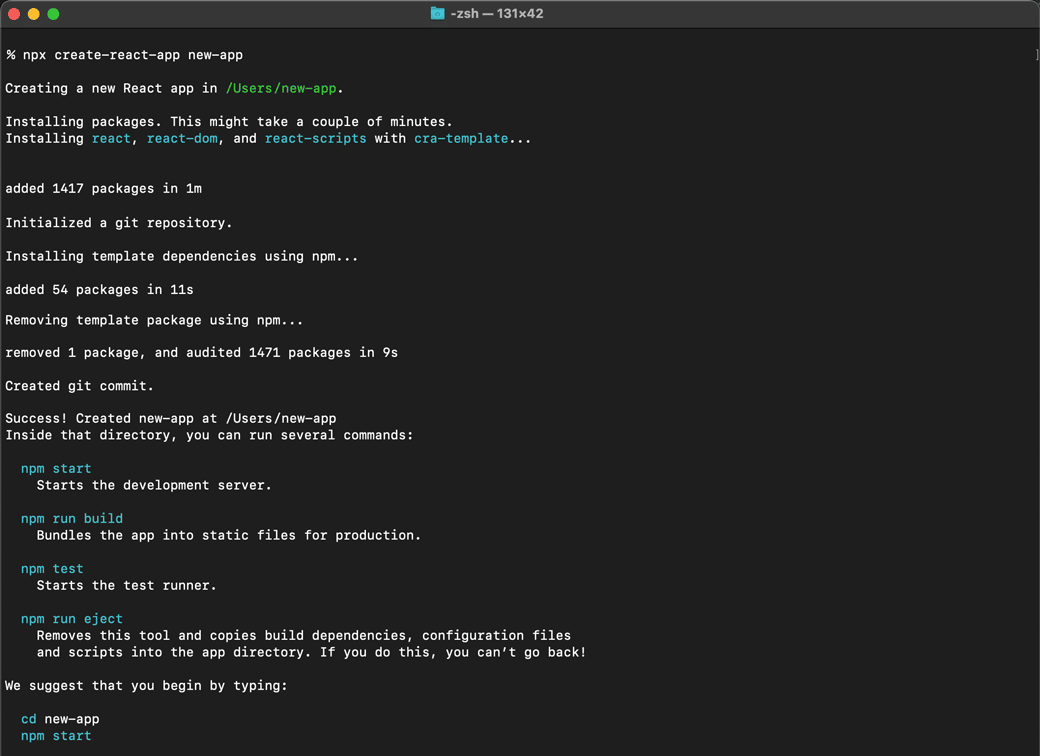
Whereas each make it straightforward to start, do not forget that Subsequent.js is constructed on high of React. So, you may’t study Subsequent.js with out first studying React and understanding the way it works. Thankfully, React boasts a delicate studying curve and is nice for learners.
It’s additionally vital to notice that React is comparatively unstructured. It’s essential to set up and arrange a React router and determine deal with information fetching, picture optimization, and code-splitting. This setup requires you to put in and configure further libraries and instruments.
In contrast, Subsequent.js comes with these instruments pre-installed and pre-configured. With Subsequent.js, any file added to the pages
folder routinely serves as a route. Due to this built-in help, Subsequent.js is less complicated to work with day by day, enabling you to begin coding your utility’s logic instantly.
Subsequent.js and React Options
As a result of Subsequent.js is predicated on React, the 2 share some options. Nevertheless, Subsequent.js goes a step additional and contains further options like routing, code-splitting, pre-rendering, and API help proper out of the field. These are options that you’d have to configure your self when utilizing React.
Information Fetching
React renders information on the shopper aspect. The server sends static recordsdata to the browser, after which the browser fetches the information from APIs to populate the appliance. This course of reduces app efficiency and offers a poor consumer expertise for the reason that app masses slowly. Subsequent.js solves this downside by way of pre-rendering.
With pre-rendering, the server makes the mandatory API calls and fetches the information earlier than sending the appliance to the browser. As such, the browser receives ready-to-render internet pages.
Pre-rendering can confer with static website technology (SSG) or server-side rendering (SSR). In SSG, the HTML pages are generated at construct time and reused for a number of requests. Subsequent.js can generate HTML pages with or with out information.
Beneath is an instance of how Subsequent.js generates pages with out information:
operate App() {
return <div>Welcome</div>
}
export default App
For static pages that devour exterior information, use the getStaticProps()
operate. When you export getStaticProps()
from a web page, Subsequent.js will pre-render the web page utilizing the props it returns. This operate all the time runs on the server, so use getStaticProps()
when the information the web page makes use of is out there at construct time. For instance, you need to use it to fetch weblog posts from a CMS.
const Posts= ({ posts }) => {
return (
<div className={types.container}>
{posts.map((put up, index) => (
// render every put up
))}
</div>
);
};
export const getStaticProps = async () => {
const posts = getAllPosts();
return {
props: { posts },
};
};
In conditions the place the web page paths rely on exterior information, use the getStaticPaths()
operate. So, to create a path based mostly on the put up ID, export the getStaticPaths()
from the web page.
For instance, you would possibly export getStaticPaths()
from pages/posts/[id].js as proven beneath.
export getStaticPaths = async() => {
// Get all of the posts
const posts = await getAllPosts()
// Get the paths you wish to pre-render based mostly on posts
const paths = posts.map((put up) => ({
params: { id: put up.id },
}))
return { paths, fallback: false }
}
getStaticPaths()
is usually paired with getStaticProps()
. On this instance, you’d use getStaticProps()
to fetch the small print of the ID within the path.
export const getStaticProps = async ({ params }) => {
const put up = await getSinglePost(params.id);
return {
props: { put up }
};
};
In SSR, information is fetched on the requested time and despatched to the browser. To make use of SSR, export the getServerSide()
props operate from the web page you wish to render. The server calls this operate on each request, which makes SSR helpful for pages that devour dynamic information.
As an example, you need to use it to fetch information from a information API.
const Information = ({ information }) => {
return (
// render information
);
};
export async operate getServerSideProps() {
const res = await fetch(`https://app-url/information`)
const information = await res.json()
return { props: { information } }
}
The information is fetched on each request and handed to the Information part through props.
Code Splitting
Code splitting is dividing code into chunks that the browser can load on demand. It reduces the quantity of code despatched to the browser in the course of the preliminary load, because the server sends solely what the consumer wants. Bundlers like Webpack, Rollup, and Browserify help code-splitting in React.
Subsequent.js helps code-splitting out of the field.
With Subsequent.js, every web page is code-split, and including pages to the appliance doesn’t improve the bundle measurement. Subsequent.js additionally helps dynamic imports, which lets you import JavaScript modules and cargo them dynamically throughout runtime. Dynamic imports contribute to quicker web page speeds as a result of bundles are lazy-loaded.
For instance, within the Dwelling part beneath, the server is not going to embody the hero part within the preliminary bundle.
const DynamicHero = dynamic(() => import('../parts/Hero'), {
suspense: true,
})
export default operate Dwelling() {
return (
<Suspense fallback={`Loading...`}>
<DynamicHero />
</Suspense>
)
}
As a substitute, the suspense’s fallback factor shall be rendered earlier than the hero part is loaded.
API Help in Subsequent.js vs React
The Subsequent.js API routing characteristic lets you write backend and frontend code in the identical codebase. Any web page saved within the /pages/api/ folder is mapped to the /api/* route, and Subsequent.js treats it like an API endpoint.
For instance, you may create a pages/api/consumer.js API route that returns the present consumer’s title like this:
export default operate consumer(req, res) {
res.standing(200).json({ username: 'Jane' });
}
If you happen to go to the https://app-url/api/consumer URL, you will note the username object.
{
username: 'Jane'
}
API routes are useful whenever you wish to masks the URL of a service you might be accessing or wish to preserve atmosphere variables a secret with out coding a whole backend utility.
Efficiency
Subsequent.js is undoubtedly superior in its capacity to create better-performing apps with a simplified course of. SSR and SSG Subsequent.js functions carry out higher than client-side rendering (CSR) React functions. By fetching information on the server and sending all the pieces the browser must render, Subsequent.js eliminates the necessity for a data-fetch request to APIs. This implies quicker load occasions.
Moreover, as a result of Subsequent.js helps client-side routing. The browser doesn’t must fetch information from the server every time a consumer navigates to a different route. Moreover, the Subsequent.js picture part permits automated picture optimization. Photos load solely after they enter the viewport. The place potential, Subsequent.js additionally serves photos in fashionable codecs like WebP.
Subsequent.js additionally offers font optimizations, sensible route prefetching, and bundling optimizations. These optimizations are usually not routinely out there in React.
Help
As a result of React has been round for longer than Subsequent.js, it has a extra in depth neighborhood. Nevertheless, many React builders are adopting Subsequent.js, in order that neighborhood is rising steadily. Builders are extra simply discovering current options to issues they encounter somewhat than having to construct options from scratch.
Subsequent.js additionally options wonderful documentation with complete examples which might be straightforward to grasp. Regardless of its recognition, React documentation will not be as navigable.
Abstract
Selecting Subsequent.js or React comes right down to an utility’s necessities.
Subsequent.js enhances React’s capabilities by offering construction and instruments that enhance efficiency. These instruments, like routing, code-splitting, and picture optimization, are constructed into Subsequent.js, which means builders don’t must configure something manually. Thanks to those options, Subsequent.js is simple to make use of, and builders can start coding the enterprise logic instantly.
Due to the totally different rendering choices, Subsequent.js is appropriate for server-side rendered functions or functions that mix static technology and Node.js server-side rendering. Additionally, due to the optimization characteristic supplied by Subsequent.js, it’s good for websites that have to be quick, like ecommerce shops.
React is a JavaScript library that may make it easier to create and scale strong front-end functions. Its syntax can also be simple, particularly for builders with a JavaScript background. Moreover, you have got management over the instruments you utilize in your utility and the way you configure them.
Planning your individual world-dominating utility? Dig into Kinsta’s strategy to Node.js utility internet hosting for companies supporting React and Subsequent.js.
Get all of your functions, databases and WordPress websites on-line and underneath one roof. Our feature-packed, high-performance cloud platform contains:
- Straightforward setup and administration within the MyKinsta dashboard
- 24/7 knowledgeable help
- The most effective Google Cloud Platform {hardware} and community, powered by Kubernetes for max scalability
- An enterprise-level Cloudflare integration for velocity and safety
- World viewers attain with as much as 35 information facilities and 275 PoPs worldwide
Take a look at it your self with $20 off your first month of Software Internet hosting or Database Internet hosting. Discover our plans or speak to gross sales to search out your greatest match.