R Core programming ideas for knowledge science defined with code
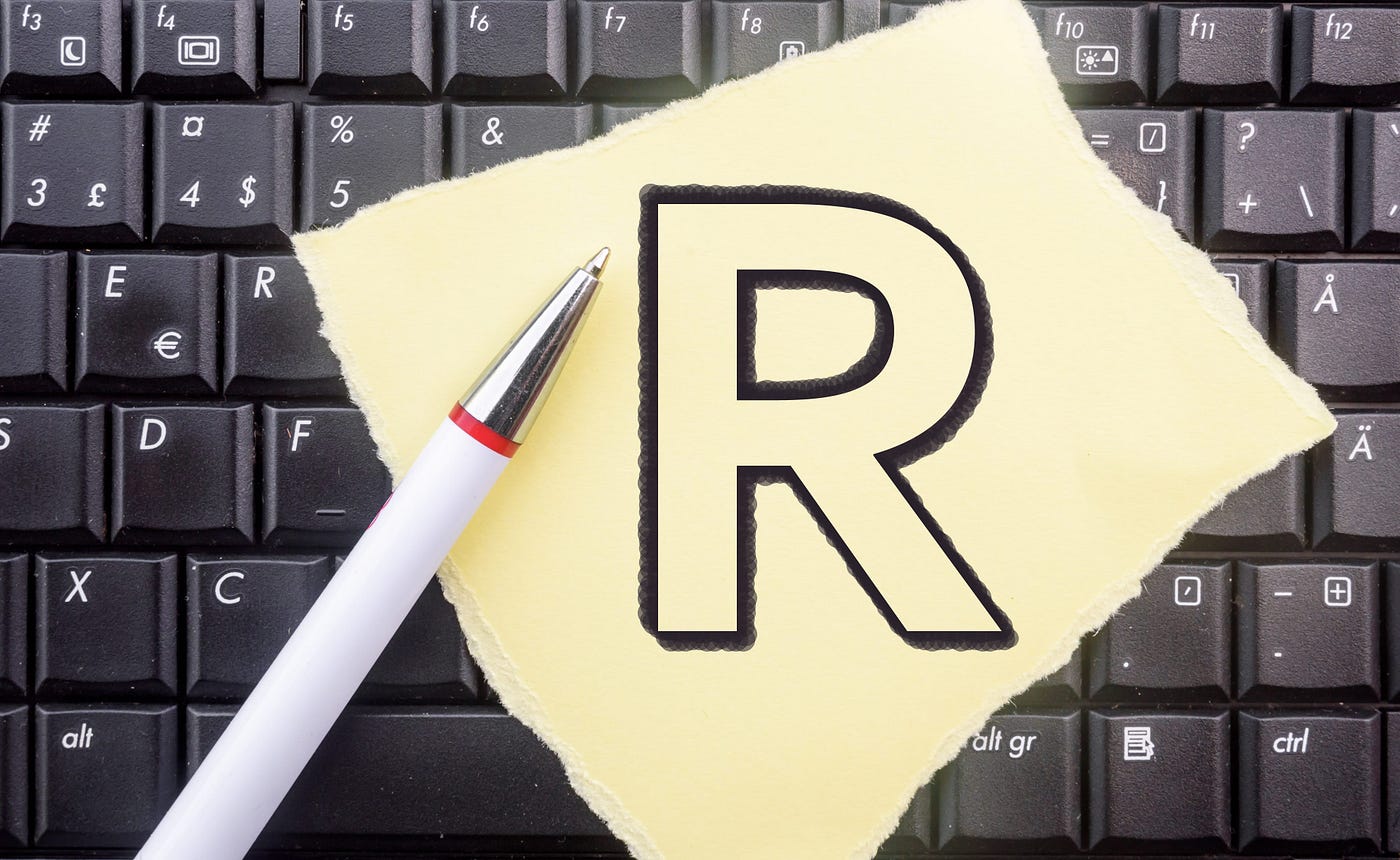
Should you’re all for studying knowledge science, you must study R. R is a programming language that excels at statistical evaluation and visualizations.
However that’s not all! With R, we are able to do knowledge evaluation, apply machine studying algorithms, and do many different knowledge science duties we might do with different programming languages utilized in knowledge science like Python.
As an alternative of selecting between Python and R for knowledge science, why not take one of the best of each worlds and turn out to be a bilingual knowledge scientist? On the finish of the day, a brand new programming language like R will open totally different job alternatives for you (even in the event you already know Python).
On this information, we’ll study some R core programming ideas each knowledge scientist ought to know. You possibly can consider this information as your first step to studying R for knowledge science!
Desk of Contents
1. R Variables
2. R Information Sorts
3. R Vectors
4. R If Assertion
5. R Whereas Loop
6. R For Loop
7. R Capabilities
In R we use variables to retailer values resembling numbers and characters. To assign a price to a variable, we use the <-
in R.
Let’s create a primary message that claims “I like R” and retailer it in a variable known as message1
.
message1 <- "I like"
Now we are able to create as many variables as we would like in R and even apply capabilities to them. For instance, we are able to concatenate 2 messages in R utilizing the paste
operate.
Let’s create a variable message2
with the worth “R programming” and concatenate it to message1
utilizing the paste
operate.
> message1 <- "I like"
> message2 <- "R programming"> paste(message1, message2)
[1] "I like R programming"
We are able to even assign the output to a brand new variable message3
.
message3 <- paste(message1, message2)
In R we use variables to retailer several types of values resembling numbers, characters, and extra. Every variable in R has a knowledge kind. Listed here are the commonest knowledge sorts within the R Programming language.
- Integer: Actual values with out decimal factors. The suffix
L
is used to specify integer knowledge. - Numeric: Set of all actual numbers
- Complicated: Purely imaginary values in R. The suffix
i
is used to specify the imaginary half. - Character: It’s used to specify character or string values (collection of characters) in a variable. Single quotes
''
or double quotes""
are used to characterize strings. - Logical: Also called boolean knowledge kind, it could possibly have
TRUE
orFALSE
values.
Right here’s the illustration of every knowledge kind in R.
x <- 1L # Integer
y <- 1.5 # Numeric
z <- 2i # Complicated
message <- "Hello!" # character
is_cold <- TRUE # logical
To examine the info kind of a variable, we use class
in R.
> message <- "Hello!"
> class(message)
[1] "character"
As we are able to see, the info kind of message
is character.
In R, a vector is a sequence of parts that share the identical knowledge kind. You possibly can really embody parts with totally different knowledge sorts inside a vector, however ultimately, they’ll be transformed to the identical knowledge kind.
To create a vector we use the c()
operate. Let’s create a vector named nations and acquire its knowledge kind.
> nations <- c(‘United States’, ‘India’, ‘China’, ‘Brazil’)
> class(nations)
[1] "character"
We’ve simply created a personality vector just because all the weather of the vector are strings.
Vector names
We are able to additionally add vector names to every ingredient of our present nations
vector. Let’s create a brand new inhabitants
vector after which add nations
as its vector identify.
> inhabitants <- c(329.5, 1393.4, 1451.5, 212.6)
> names(inhabitants) <- nations> inhabitants
United States India China Brazil
329.5 1393.4 1451.5 212.6
Vector indexing
We are able to receive a selected ingredient in a vector by indexing. Every merchandise in a vector has an index (aka place). In contrast to different programming languages, indexing in R begins at 1.
In R, to entry a component by its index we use sq. brackets []
. Let’s see just a few examples utilizing the nations
vector we’ve created earlier than.
> nations[1]
[1] "United States"> nations[4]
[1] "Brazil"
We are able to additionally index a number of parts utilizing the c()
operate inside sq. brackets and even use the vector names as a substitute of the index place.
> nations[c(1,4)]
[1] "United States" "Brazil"> inhabitants[c("United States", "China")]
United States China
329.5 1451.5
Vector slicing
Vector slicing means accessing a portion of a vector. A slice is a subset of parts. The notation is the next:
vector[start:stop]
the place “begin” represents the index of the primary ingredient, and cease represents the ingredient to cease at (together with it within the slice).
Let’s see some examples:
> inhabitants[1:3]
United States India China
329.5 1393.4 1451.5
Nice! We’ve obtained the weather between indexes 1 and three.
Filtering
We are able to filter out some parts of a vector utilizing comparisons. The syntax to make use of is just like indexing, however as a substitute of typing the index inside sq. brackets []
, we make the comparability utilizing a logical operator.
Let’s filter out nations with a inhabitants lower than 300 million.
> inhabitants[population>300]
United States India China
329.5 1393.4 1451.5
As we are able to see, the nation Brazil with a inhabitants of 212 million was filtered out from our vector.
Similar to in every other programming language, the if
assertion is quite common in R. We use it to resolve whether or not an announcement(s) will likely be executed.
Right here’s the syntax of the if
assertion in R:
if (condition1) {
statement1
} else if (condition2) {
statement2
} else {
statement3
}
Let’s examine how this works with an instance. The code beneath will output a message primarily based on the top
variable.
top <- 1.9if (top > 1.8){
print("You are tall")
} else if(top > 1.7){
print("You are common")
} else {
print("You are quick")
}
What the code is saying is “in case your top is above 1.8m, you’re tall; if the peak is between 1.7 and 1.8, you’re common; and if the peak is 1.7 or beneath, you’re quick”
The whereas
loop permits us to repeat a selected block of code so long as the desired situation is happy.
Right here’s the syntax of the whereas
loop in R:
whereas (situation) {
<code>
}
Let’s see the way it works with the next instance.
x <- 5whereas(x<10){
print(x)
x <- x+1
}
If we run the code above, the output would be the following:
[1] 5
[1] 6
[1] 7
[1] 8
[1] 9
We acquired values till the quantity 9 as a result of after that the situation x<10 was unhappy, so the loop broke.
We are able to additionally use the break
key phrase to interrupt out of a loop. To take action, we often mix it with the if
assertion. Let’s see an instance.
x <- 5whereas(x<10){
print(x)
x <- x+1
if (x==8){
print("x is the same as 8. Break loop!")
break
}
}
The output would be the following:
[1] 5
[1] 6
[1] 7
[1] "x is the same as 8. Break loop!"
On this case, the whereas
loop broke when x reached the worth of 8 due to the brand new situation we created.
Probably the most frequent loops in any programming language is the for
loop. The for loop permits us to iterate over objects of a sequence (like our vectors in R) and carry out an motion on every merchandise.
Right here’s the syntax of the for
loop in R:
for (val in sequence)
{
<code>
}
Let’s loop by means of the nations
vector that we created earlier than and print every merchandise.
> nations <- c('United States', 'India', 'China', 'Brazil')> for (i in nations){
+ print(i)
+ }[1] "United States"
[1] "India"
[1] "China"
[1] "Brazil"
We are able to additionally use the for loop and if assertion collectively to carry out an motion to solely sure parts.
For instance, let’s loop by means of the nations
vector however now solely print the ingredient “India”
> for (i in nations){
+ if (i=="India"){
+ print(i)
+ }
+ }[1] "India"
R has totally different built-in capabilities that assist us carry out a selected motion. Thus far we’ve seen the paste
and c
operate, however there are a lot of others. To call just a few:
sum() -> returns sum
rnorm() -> draw random samples from a distribution
sqrt() -> returns the sq. root of an enter
tolower() -> converts the string into decrease case
However that’s not all! We are able to additionally create our personal operate in R. We’ve to observe the syntax beneath.
function_name <- operate(<params>){
<code>
return(<output>)
}
Let’s create a operate that converts the temperature from Fahrenheit to Celsius.
fahrenheit_to_celsius <- operate(temp_f){
temp_c <- (temp_f - 32) * 5 / 9
return(temp_c)
}
Now if we name the operate fahrenheit_to_celsius()
we’ll convert the temperature from Fahrenheit to Celsius.
> fahrenheit_to_celsius(100)
[1] 37.77778