Customized fields present a technique to assign additional data to web site content material. These bits of knowledge are normally generally known as metadata.
Metadata is details about data. Within the case of WordPress, it’s data related to posts, customers, feedback and phrases.
Given the many-to-one relationship of metadata in WordPress, your choices are pretty limitless. You’ll be able to have as many meta choices as you want, and you may retailer absolutely anything in there.
—Fb
Listed below are some examples of metadata you possibly can connect to a submit utilizing customized fields:
- The geographic coordinates of a spot or actual property
- The date of an occasion
- The ISBN or creator of a e book
- The temper of the day of the creator of the submit
And there are loads extra.
Out of the field, WordPress doesn’t present a simple approach so as to add and handle customized fields. Within the Basic Editor, customized fields are displayed in a field positioned on the backside of the web page, beneath the submit editor.
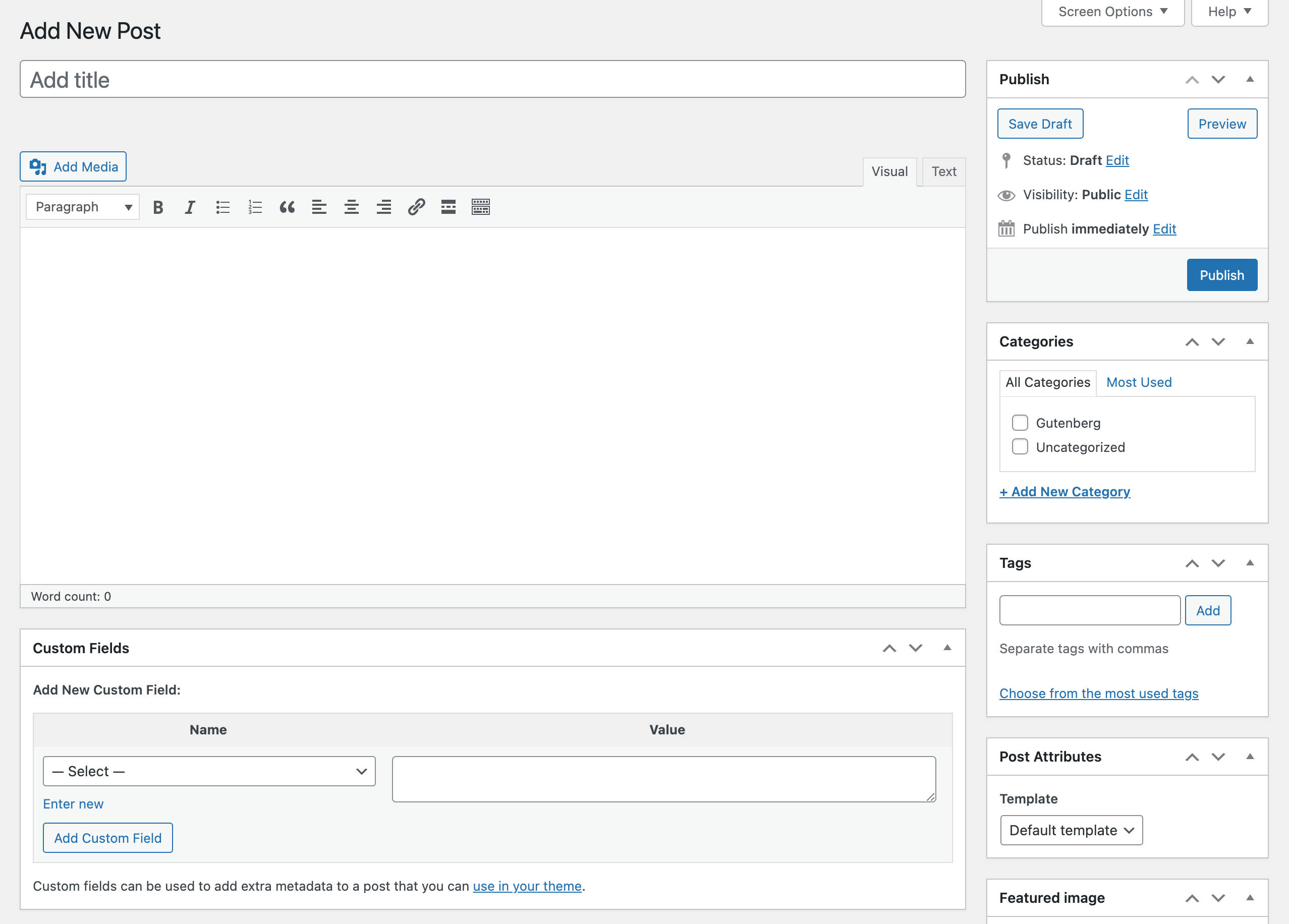
In Gutenberg, customized fields are disabled by default, however you possibly can show them by deciding on the corresponding merchandise in submit settings.
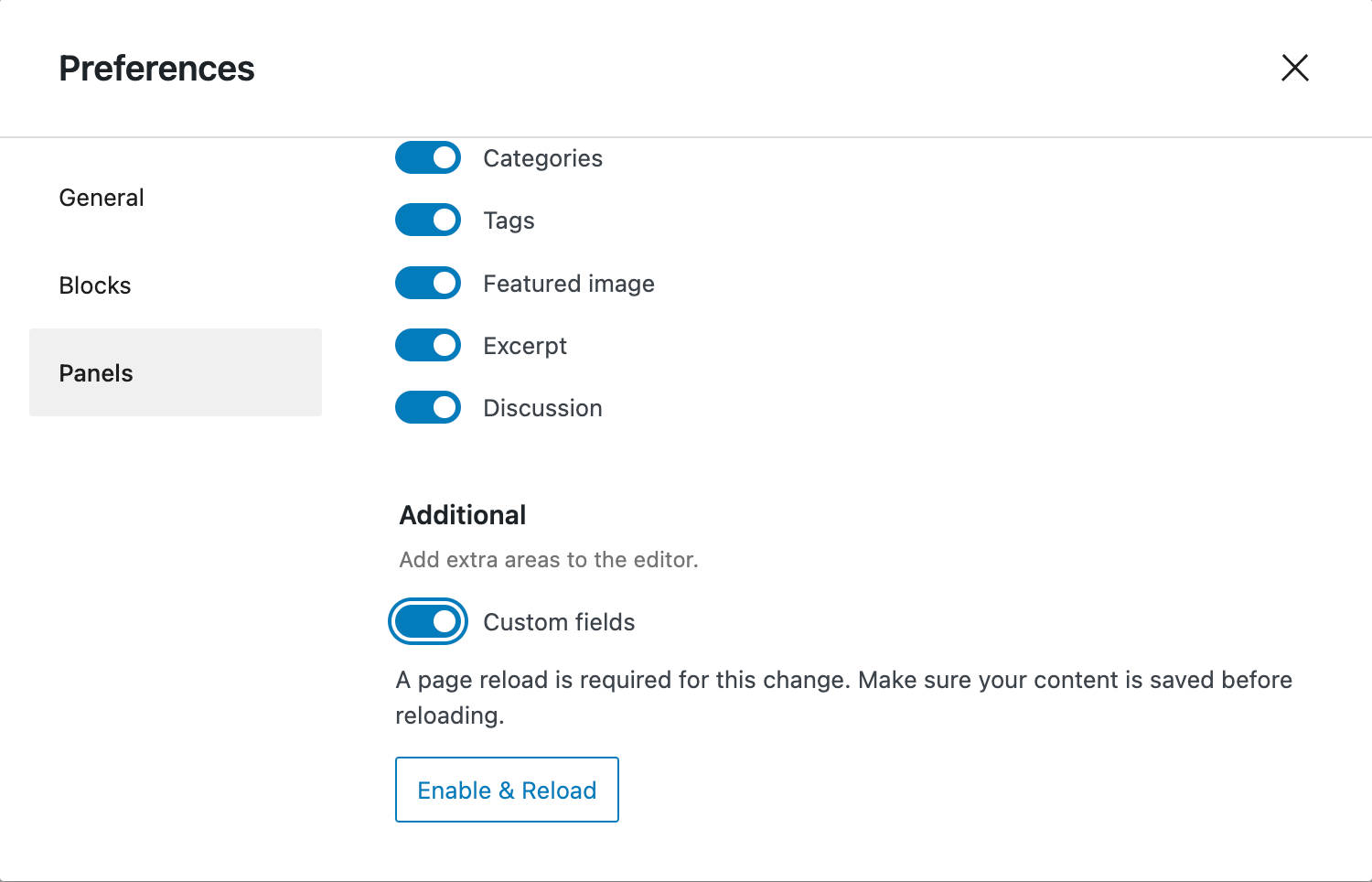
Sadly, there isn’t any technique to show metadata on the frontend with out utilizing a plugin or getting your fingers soiled with code.
If you happen to’re a consumer, you’ll discover a number of glorious plugins doing the job for you on the market. However when you’re a developer and need to get extra out of WordPress customized fields, combine them seamlessly into the block editor, and show them on the frontend of your WordPress web site utilizing a customized Gutenberg block, then you definately’re in the proper place.
So, when you’re questioning what’s one of the simplest ways to make use of WordPress customized fields each in Gutenberg and the Basic Editor for WordPress builders, the fast reply is “making a plugin that works for each the Basic Editor and Gutenberg”.
However don’t fear an excessive amount of. If making a plugin to handle customized fields in each editors may very well be slightly tough, we’ll attempt to make the method as easy as attainable. When you perceive the ideas we’ll focus on on this article, you’ll achieve the abilities wanted to handle customized meta fields in Gutenberg and construct every kind of internet sites.
Observe: Earlier than doing something, be sure you have an up-to-date model of Node.js in your pc
That having been stated, right here is our rundown:
Create a Block Plugin With the Official create-block Device
Step one is to create a brand new plugin with all of the recordsdata and dependencies wanted to register a brand new block sort. The block plugin will will let you simply construct a customized block sort for managing and displaying customized metadata.
To create a brand new block sort, we’ll use the official create-block instrument. For an in depth overview of methods to use the create-block instrument, take a look at our earlier article about Gutenberg block growth.
Open your command line instrument, navigate to the plugins listing of your WordPress growth web site and run the next command:
npx @wordpress/create-block
When prompted, add the next particulars:
- The template variant to make use of for this block: dynamic
- The block slug used for identification (additionally the output folder identify): metadata-block
- The interior namespace for the block identify (one thing distinctive on your merchandise): meta-fields
- The show title on your block: Meta Fields
- The brief description on your block (elective): Block description
- The dashicon to make it simpler to establish your block (elective): e book
- The class identify to assist customers browse and uncover your block: widgets
- Do you need to customise the WordPress plugin? Sure/No
Let’s take a second to overview these particulars and attempt to perceive the place they’re used.
- The block slug used for identification defines the plugin’s folder identify and textdomain
- The interior namespace for the block identify defines the block inner namespace and operate prefix used all through the plugin’s code.
- The show title on your block defines the plugin identify and the block identify used within the editor interface.
The setup might take a few minutes. When the method is accomplished, you’ll get a listing of the obtainable instructions.
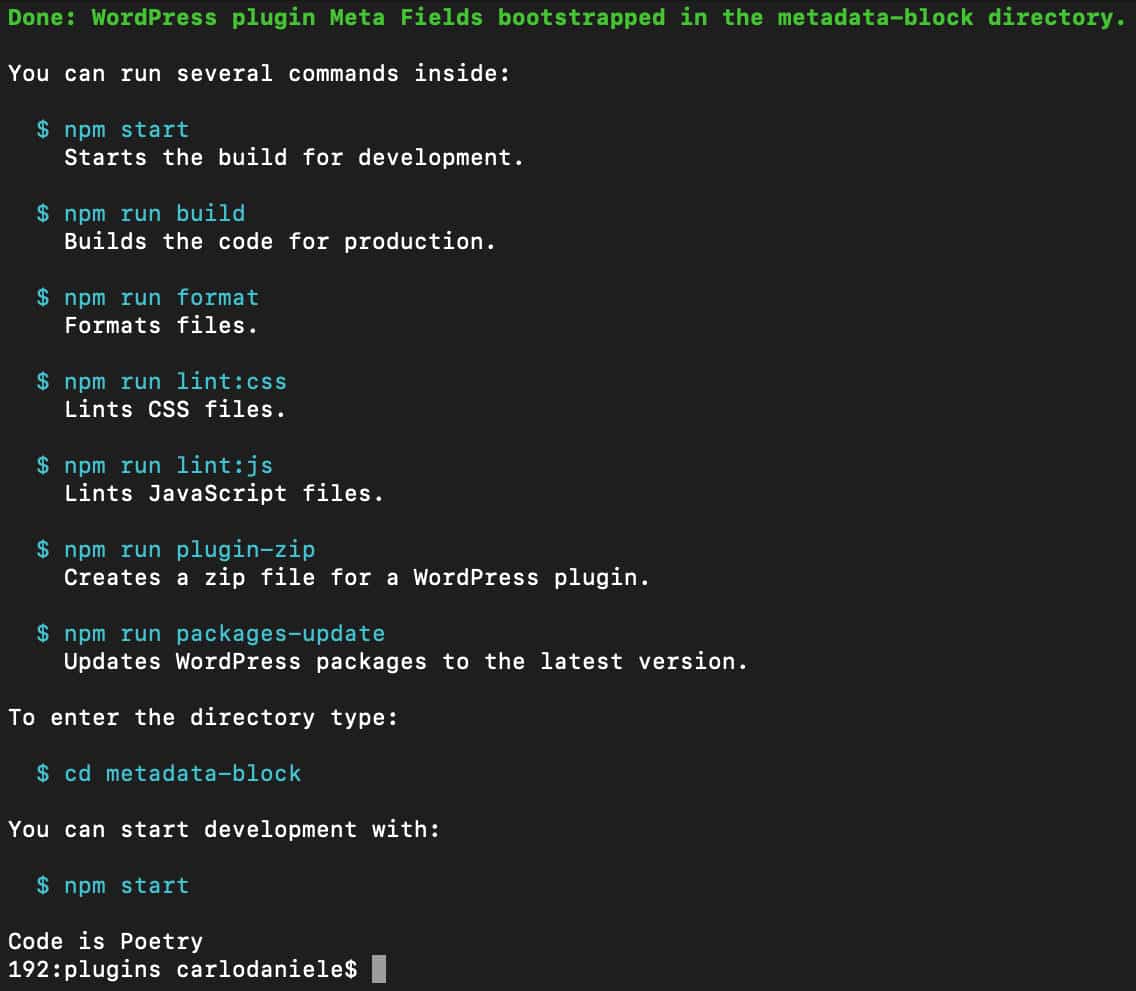
Earlier than shifting on to the subsequent part, in your command line instrument, navigate to your plugin’s folder and run the next instructions:
cd metadata-block
npm begin
You’re able to construct your code. The subsequent step is to edit the primary PHP file of the plugin to construct a meta field for the Basic Editor.
So, earlier than shifting on to the subsequent part, set up and activate the Basic Editor plugin.
Then, open the Plugins display screen and activate the brand new Meta Fields plugin.
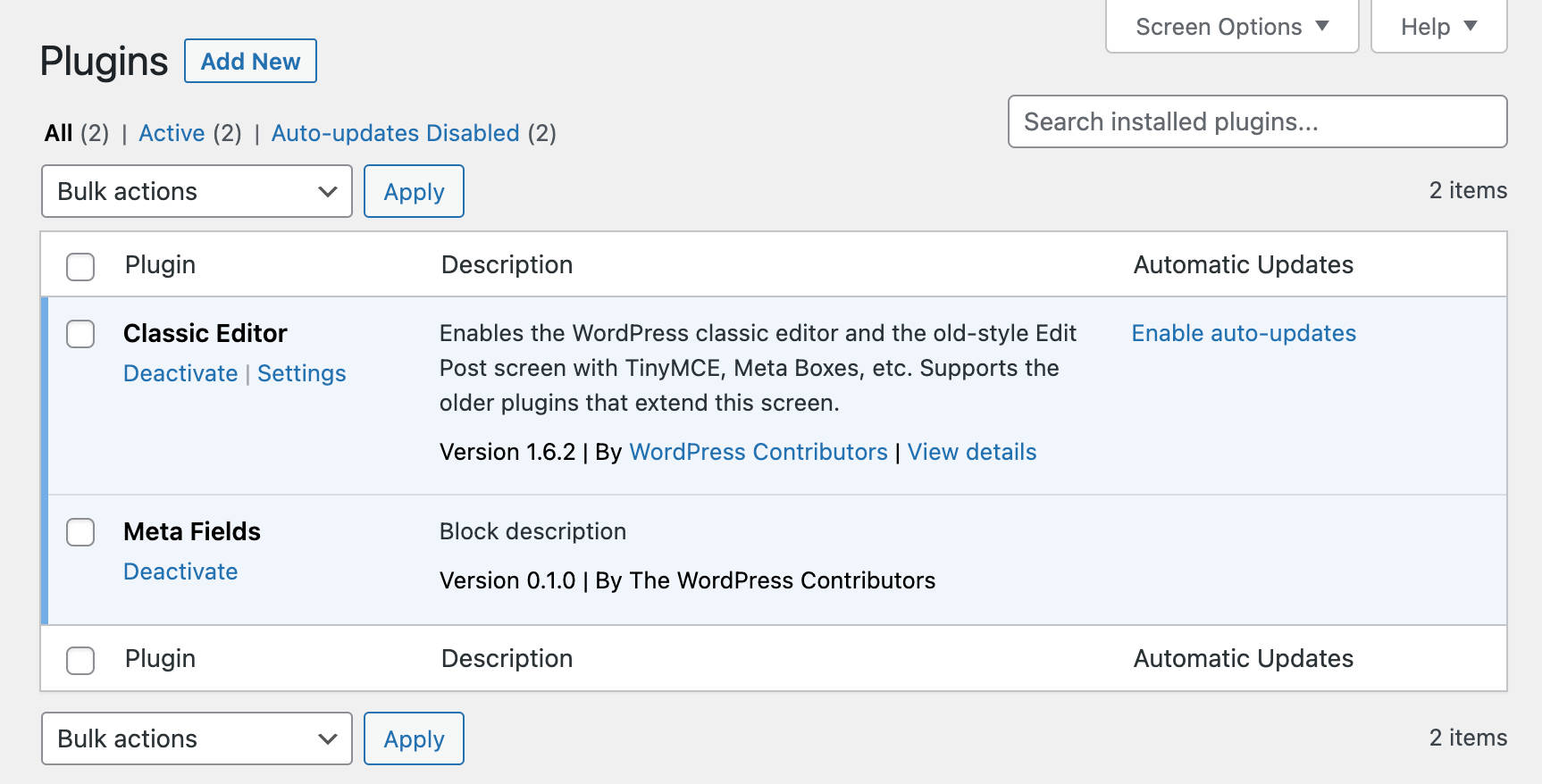
Add a Meta Field to the Basic Editor
Within the context of the Basic Editor, a meta field is a container holding kind components to sort in particular bits of knowledge, such because the submit creator, tags, classes, and many others.
Along with the built-in meta containers, plugin builders can add any variety of customized meta containers to incorporate HTML kind components (or any HTML content material) the place plugin customers can enter plugin-specific knowledge.
The WordPress API offers helpful capabilities to simply register customized meta containers to incorporate all of the HTML components your plugin must work.
To get began, append the next code to the PHP file of the plugin you’ve simply created:
// register meta field
operate meta_fields_add_meta_box(){
add_meta_box(
'meta_fields_meta_box',
__( 'Guide particulars' ),
'meta_fields_build_meta_box_callback',
'submit',
'facet',
'default'
);
}
// construct meta field
operate meta_fields_build_meta_box_callback( $submit ){
wp_nonce_field( 'meta_fields_save_meta_box_data', 'meta_fields_meta_box_nonce' );
$title = get_post_meta( $post->ID, '_meta_fields_book_title', true );
$creator = get_post_meta( $post->ID, '_meta_fields_book_author', true );
?>
<div class="inside">
<p><sturdy>Title</sturdy></p>
<p><enter sort="textual content" id="meta_fields_book_title" identify="meta_fields_book_title" worth="<?php echo esc_attr( $title ); ?>" /></p>
<p><sturdy>Creator</sturdy></p>
<p><enter sort="textual content" id="meta_fields_book_author" identify="meta_fields_book_author" worth="<?php echo esc_attr( $creator ); ?>" /></p>
</div>
<?php
}
add_action( 'add_meta_boxes', 'meta_fields_add_meta_box' );
The add_meta_box
operate registers a brand new meta field, whereas the callback operate builds the HTML to be injected into the meta field. We received’t dive deeper into this matter as a result of it’s past the scope of our article, however you’ll discover all the main points you want right here, right here and right here.
The subsequent step is to create a operate that saves the info entered by the submit creator anytime the save_post
hook is triggered (see Developer Sources):
// save metadata
operate meta_fields_save_meta_box_data( $post_id ) {
if ( ! isset( $_POST['meta_fields_meta_box_nonce'] ) )
return;
if ( ! wp_verify_nonce( $_POST['meta_fields_meta_box_nonce'], 'meta_fields_save_meta_box_data' ) )
return;
if ( outlined( 'DOING_AUTOSAVE' ) && DOING_AUTOSAVE )
return;
if ( ! current_user_can( 'edit_post', $post_id ) )
return;
if ( ! isset( $_POST['meta_fields_book_title'] ) )
return;
if ( ! isset( $_POST['meta_fields_book_author'] ) )
return;
$title = sanitize_text_field( $_POST['meta_fields_book_title'] );
$creator = sanitize_text_field( $_POST['meta_fields_book_author'] );
update_post_meta( $post_id, '_meta_fields_book_title', $title );
update_post_meta( $post_id, '_meta_fields_book_author', $creator );
}
add_action( 'save_post', 'meta_fields_save_meta_box_data' );
Once more, take a look at the net documentation for particulars. Right here we’ll simply level out the underscore character (_
) previous the meta key. This tells WordPress to cover the keys of those customized fields from the record of customized fields obtainable by default and makes your customized fields seen solely in your customized meta field.
The picture beneath exhibits what the customized meta field appears to be like like within the Basic Editor:
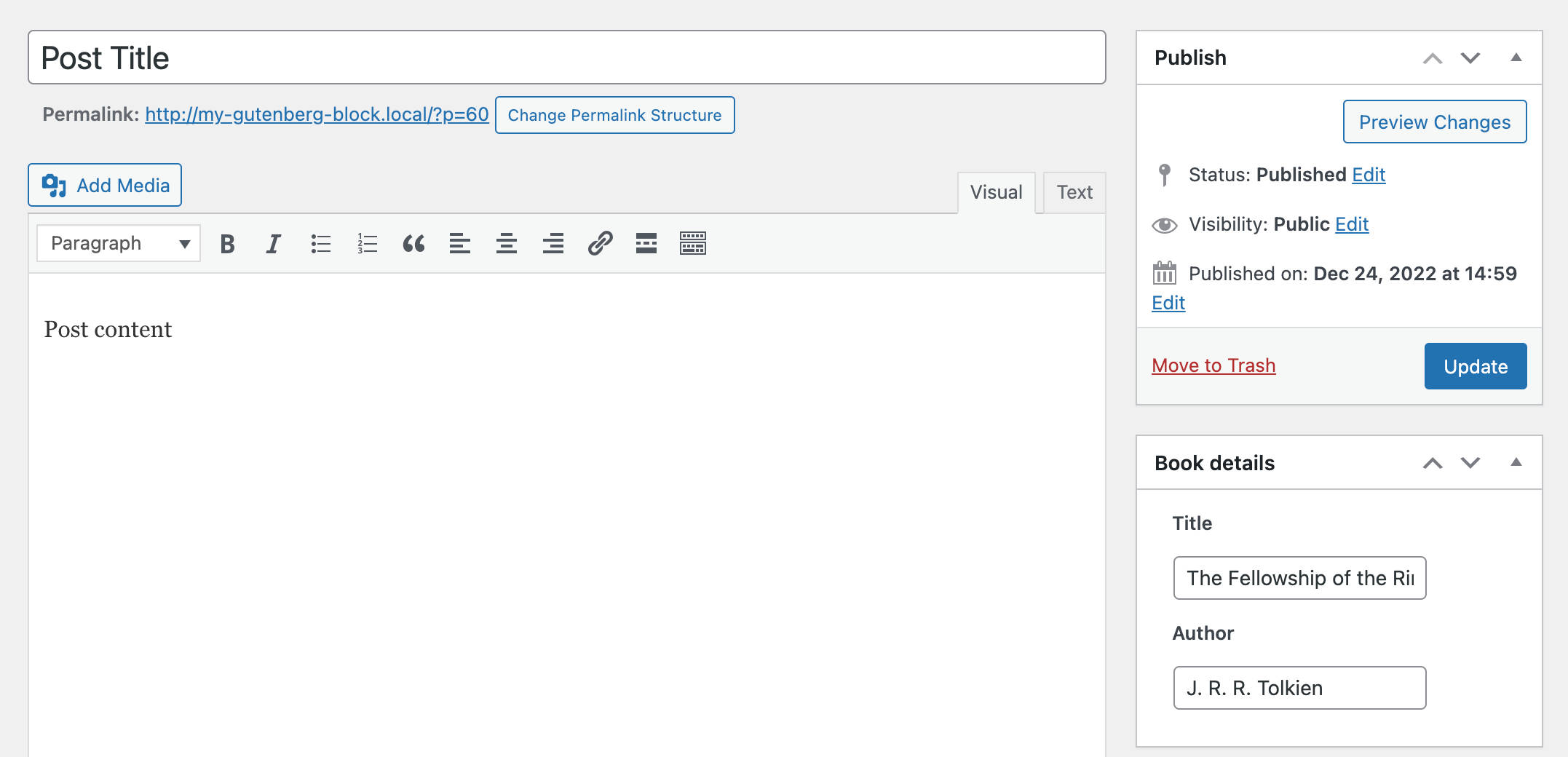
Now, when you disable the Basic Editor plugin and test what occurs within the block editor, you’ll see that the meta field nonetheless seems and works, however not precisely in the best way you may anticipate.
Our purpose is to create a system for managing metadata connected to weblog posts or customized submit sorts that integrates seamlessly inside the block editor. Because of this, the code proven thus far will solely be wanted to make sure backward compatibility with the Basic Editor.
So, earlier than shifting on, we’ll inform WordPress to take away the customized meta field from the block editor by including the __back_compat_meta_box
flag to the add_meta_box
operate (see additionally Meta Field Compatibility Flags and Backward Compatibility).
Let’s get again to the callback operate that registers the meta field and alter it as follows:
// register meta field
operate meta_fields_add_meta_box(){
add_meta_box(
'meta_fields_meta_box',
__( 'Guide particulars' ),
'meta_fields_build_meta_box_callback',
'submit',
'facet',
'default',
// conceal the meta field in Gutenberg
array('__back_compat_meta_box' => true)
);
}
Save the plugin file and return to your WordPress admin. Now, you shouldn’t see the customized meta field within the block editor anymore. If you happen to reactivate the Basic Editor as a substitute, your customized meta field will present up once more.
Add Customized Meta Fields to the Gutenberg Block Editor (Three Choices)
In our earlier articles about Gutenberg block growth, we offered detailed overviews of the editor, its elements, and methods to develop static blocks and dynamic blocks.
As we talked about, on this article we’ll take it a step additional and focus on methods to add customized meta fields to weblog posts.
There are a number of methods to retailer and use submit metadata in Gutenberg. Right here we’ll cowl the next:
Create a Customized Block To Retailer and Show Customized Meta Fields
On this part, we’ll present you methods to create and handle customized meta fields from inside a dynamic block. In accordance with the Block Editor Handbook, a submit meta area “is a WordPress object used to retailer additional knowledge a few submit” and we have to first register a brand new meta area earlier than we are able to use it.
Register Customized Meta Fields
Earlier than registering a customized meta area, you must ensure that the submit sort that can use it helps customized fields. As well as, once you register a customized meta area, you must set the show_in_rest
parameter to true
.
Now, again to the plugin file. Add the next code:
/**
* Register the customized meta fields
*/
operate meta_fields_register_meta() {
$metafields = [ '_meta_fields_book_title', '_meta_fields_book_author' ];
foreach( $metafields as $metafield ){
// Cross an empty string to register the meta key throughout all current submit sorts.
register_post_meta( '', $metafield, array(
'show_in_rest' => true,
'sort' => 'string',
'single' => true,
'sanitize_callback' => 'sanitize_text_field',
'auth_callback' => operate() {
return current_user_can( 'edit_posts' );
}
));
}
}
add_action( 'init', 'meta_fields_register_meta' );
register_post_meta
registers a meta key for the required submit sorts. Within the code above, we’ve registered two customized meta fields for all submit sorts registered in your web site that help customized fields. For extra data, see the operate reference.
As soon as performed, open the src/index.js file of your block plugin.
Register the Block Sort on the Shopper
Now navigate to the wp-content/plugins/metadata-block/src folder and open the index.js file:
import { registerBlockType } from '@wordpress/blocks';
import './model.scss';
import Edit from './edit';
import metadata from './block.json';
registerBlockType( metadata.identify, {
edit: Edit,
} );
With static blocks we’d even have seen a save
operate. On this case, the save
operate is lacking as a result of we put in a dynamic block. The content material proven on the frontend can be generated dynamically through PHP.
Construct the Block Sort
Navigate to the wp-content/plugins/metadata-block/src folder and open the edit.js file. It’s best to see the next code (feedback eliminated):
import { __ } from '@wordpress/i18n';
import { useBlockProps } from '@wordpress/block-editor';
import './editor.scss';
export default operate Edit() {
return (
<p { ...useBlockProps() }>
{ __( 'Meta Fields – whats up from the editor!', 'metadata-block' ) }
</p>
);
}
Right here you’ll add the code to generate the block to be rendered within the editor.
Step one is to import the parts and capabilities wanted to construct the block. Right here is the whole record of dependencies:
import { __ } from '@wordpress/i18n';
import { useBlockProps, InspectorControls, RichText } from '@wordpress/block-editor';
import { useSelect } from '@wordpress/knowledge';
import { useEntityProp } from '@wordpress/core-data';
import { TextControl, PanelBody, PanelRow } from '@wordpress/parts';
import './editor.scss';
If you happen to’ve learn our earlier articles, try to be acquainted with many of those import
declarations. Right here we’ll level out simply a few them:
import { useSelect } from '@wordpress/knowledge';
import { useEntityProp } from '@wordpress/core-data';
When you’ve imported these dependencies, right here is the way you’ll useSelect
and useEntityProp
within the Edit()
operate:
const postType = useSelect(
( choose ) => choose( 'core/editor' ).getCurrentPostType(),
[]
);
const [ meta, setMeta ] = useEntityProp( 'postType', postType, 'meta' );
This code offers the present postType
, an object of meta fields (meta
), and a setter operate to replace them (setMeta
).
Now substitute the present code for the Edit()
operate with the next:
export default operate Edit() {
const blockProps = useBlockProps();
const postType = useSelect(
( choose ) => choose( 'core/editor' ).getCurrentPostType(),
[]
);
const [ meta, setMeta ] = useEntityProp( 'postType', postType, 'meta' );
const bookTitle = meta[ '_meta_fields_book_title' ];
const bookAuthor = meta[ '_meta_fields_book_author' ];
const updateBookTitleMetaValue = ( newValue ) => {
setMeta( { ...meta, _meta_fields_book_title: newValue } );
};
const updateBookAuthorMetaValue = ( newValue ) => {
setMeta( { ...meta, _meta_fields_book_author: newValue } );
};
return ( ... );
}
Once more:
- We used
useSelect
to get the present submit sort. useEntityProp
returns an array of meta fields and a setter operate to set new meta values.updateBookTitleMetaValue
andupdateBookAuthorMetaValue
are two occasion handlers to save lots of meta area values.
The subsequent step is to construct the JSX (JavaScript XML) code returned by the Edit()
operate:
export default operate Edit() {
...
return (
<>
<InspectorControls>
<PanelBody
title={ __( 'Guide Particulars' )}
initialOpen={true}
>
<PanelRow>
<fieldset>
<TextControl
label={__( 'Guide title' )}
worth={ bookTitle }
onChange={ updateBookTitleMetaValue }
/>
</fieldset>
</PanelRow>
<PanelRow>
<fieldset>
<TextControl
label={ __( 'Guide creator' ) }
worth={ bookAuthor }
onChange={ updateBookAuthorMetaValue }
/>
</fieldset>
</PanelRow>
</PanelBody>
</InspectorControls>
<div { ...blockProps }>
<RichText
tagName="h3"
onChange={ updateBookTitleMetaValue }
allowedFormats={ [ 'core/bold', 'core/italic' ] }
worth={ bookTitle }
placeholder={ __( 'Write your textual content...' ) }
/>
<TextControl
label="Guide Creator"
worth={ bookAuthor }
onChange={ updateBookAuthorMetaValue }
/>
</div>
</>
);
}
The RichText
part offers a contenteditable enter, whereas TextControl
offers easy textual content fields.
We additionally created a sidebar panel containing two enter fields to make use of as a substitute of the 2 kind controls included within the block.
Save the file and return to the editor. Add the Meta Fields block from the block inserter and fill within the e book title and creator.
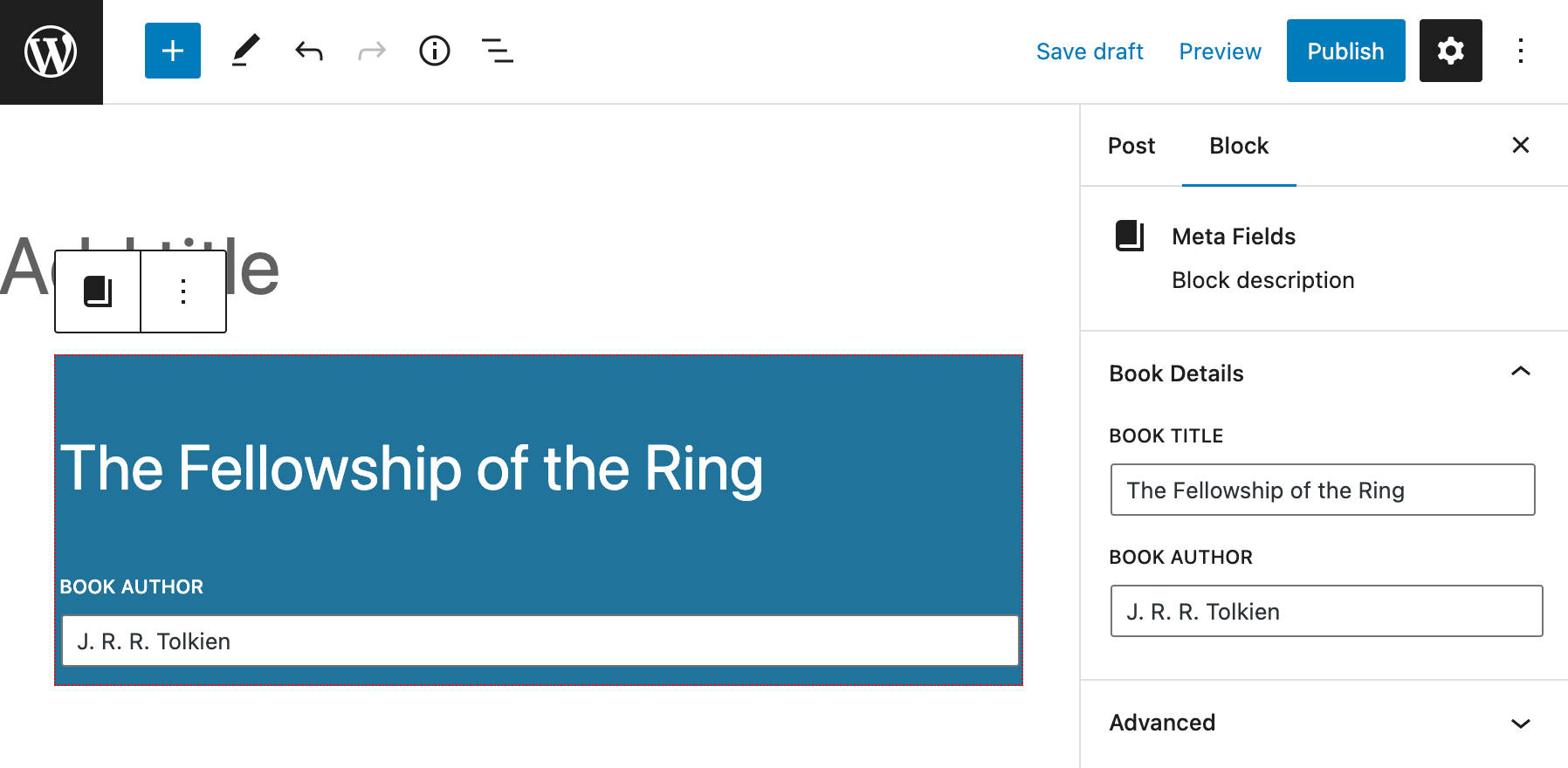
You’ll discover that everytime you change the worth of the sphere within the block, the worth within the corresponding textual content area within the sidebar may even change.
Subsequent, we’ve to create the PHP code that generates the HTML to be rendered on the frontend.
Show the Block on the Frontend
Open the primary PHP file once more in your code editor and rewrite the callback operate that generates the output of the block as follows:
operate meta_fields_metadata_block_block_init() {
register_block_type(
__DIR__ . '/construct',
array(
'render_callback' => 'meta_fields_metadata_block_render_callback',
)
);
}
add_action( 'init', 'meta_fields_metadata_block_block_init' );
operate meta_fields_metadata_block_render_callback( $attributes, $content material, $block ) {
$book_title = get_post_meta( get_the_ID(), '_meta_fields_book_title', true );
$book_author = get_post_meta( get_the_ID(), '_meta_fields_book_author', true );
$output = "";
if( ! empty( $book_title ) ){
$output .= '<h3>' . esc_html( $book_title ) . '</h3>';
}
if( ! empty( $book_author ) ){
$output .= '<p>' . __( 'Guide creator: ' ) . esc_html( $book_author ) . '</p>';
}
if( strlen( $output ) > 0 ){
return '<div ' . get_block_wrapper_attributes() . '>' . $output . '</div>';
} else {
return '<div ' . get_block_wrapper_attributes() . '>' . '<sturdy>' . __( 'Sorry. No fields obtainable right here!' ) . '</sturdy>' . '</div>';
}
}
This code is kind of self-explanatory. First, we use get_post_meta
to retrieve the values of the customized meta fields. Then we use these values to construct the block content material. Lastly, the callback operate returns the HTML of the block.
The block is prepared for use. We deliberately stored the code on this instance so simple as attainable however utilizing Gutenberg’s native parts you possibly can construct extra superior blocks and get probably the most from WordPress customized meta fields.
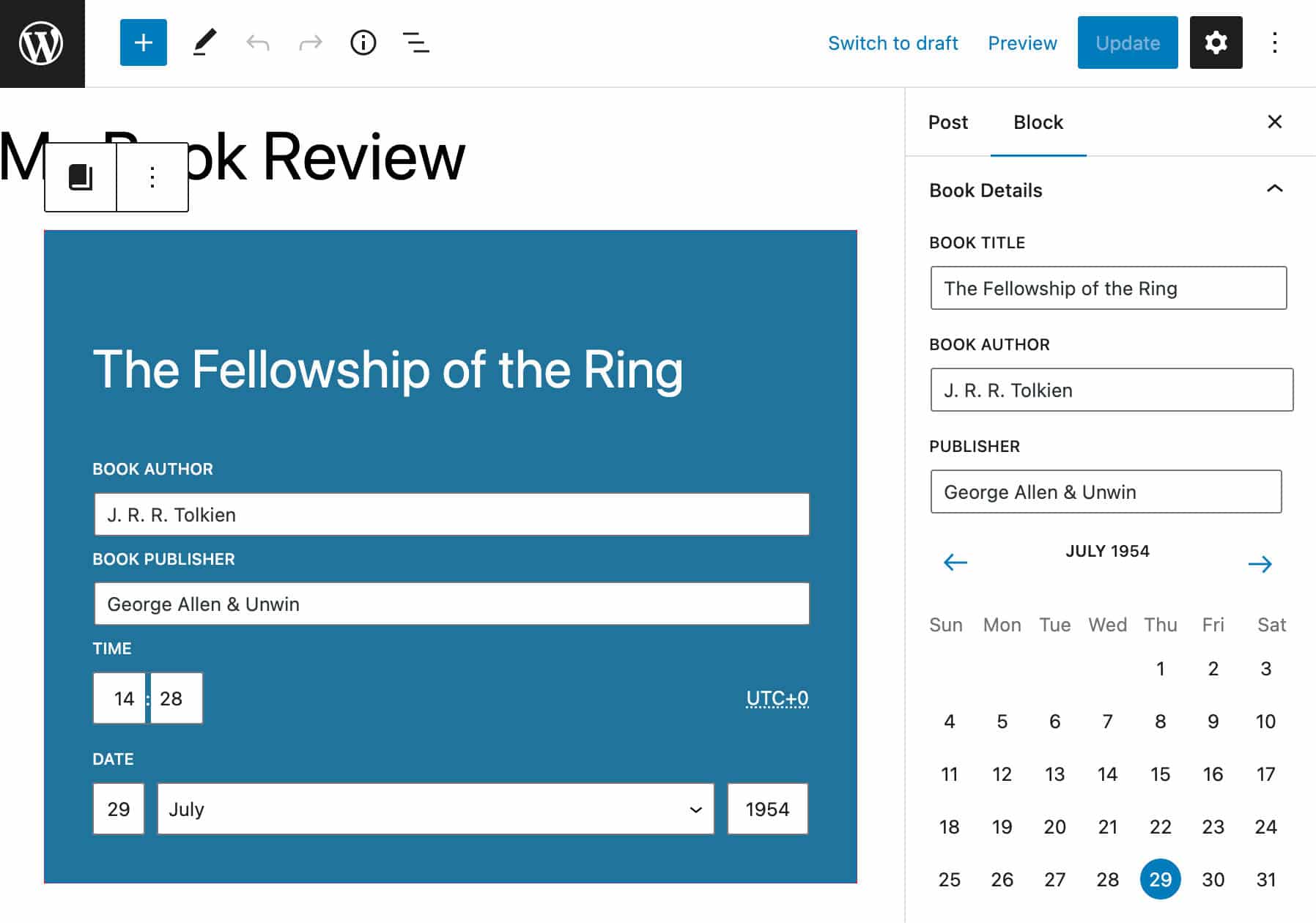
In our instance, we used h3
and p
components to construct the block for the frontend.
However you possibly can show the info in some ways. The next picture exhibits a easy unordered record of meta fields.
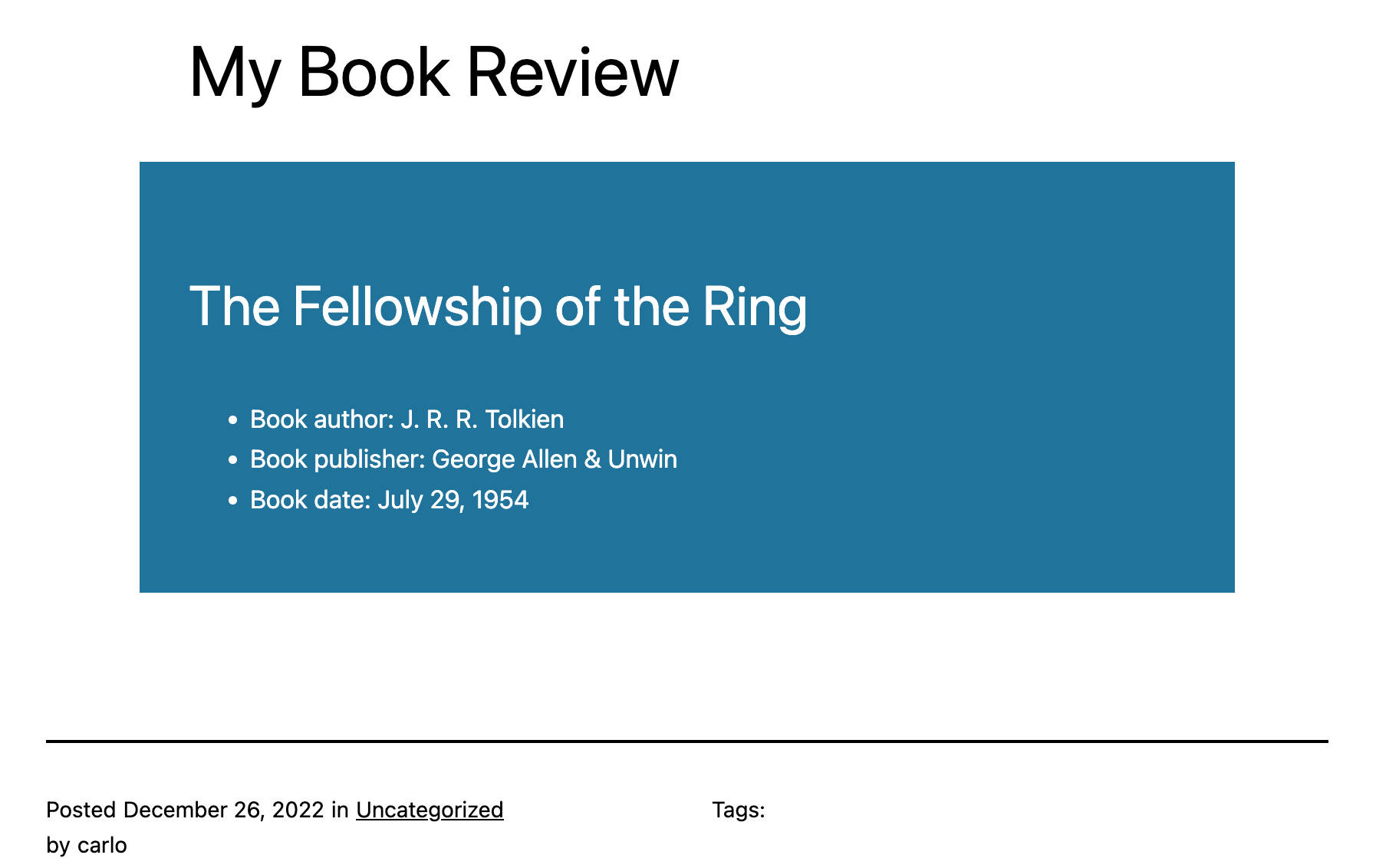
You’ll discover the whole code of this instance in this public gist.
Including a Customized Meta Field to the Doc Sidebar
The second choice is to connect customized meta fields to posts utilizing a plugin that generates a settings panel within the Doc Sidebar.
The method is fairly much like the earlier instance, besides that on this case, we received’t want a block to handle metadata. We’ll create a part to generate a panel together with a set of controls within the Doc sidebar following these steps:
- Create a brand new block plugin with create-block
- Register a customized meta field for the Basic Editor
- Register the customized meta fields in the primary plugin file through the register_post_meta() operate
- Register a plugin within the index.js file
- Construct the part utilizing built-in Gutenberg parts
Create a New Block Plugin With the create-block Device
To create a brand new block plugin, observe the steps within the earlier part. You’ll be able to create a brand new plugin or edit the scripts we constructed within the earlier instance.
Register a Customized Meta Field for the Basic Editor
Subsequent, you must register a customized meta field to make sure backward compatibility for WordPress web sites nonetheless utilizing the Basic Editor. The method is identical as described within the earlier part.
Register the Customized Meta Fields within the Most important Plugin File
The subsequent step is to register the customized meta fields in the primary plugin file through the register_post_meta()
operate. Once more, you possibly can observe the earlier instance.
Register a Plugin within the index.js File
When you’ve accomplished the earlier steps, it’s time to register a plugin within the index.js file to render a customized part.
Earlier than registering the plugin, create a parts folder contained in the plugin’s src folder. Contained in the parts folder, create a brand new MetaBox.js file. You’ll be able to select no matter identify you suppose is nice on your part. Simply ensure to observe the greatest follow for naming in React.
Earlier than shifting on, set up the @wordpress/plugins
module out of your command line instrument.
Cease the method (mac), set up the module and begin the method once more:
^C
npm set up @wordpress/plugins --save
npm begin
As soon as performed, open the index.js file of your plugin and add the next code.
/**
* Registers a plugin for including objects to the Gutenberg Toolbar
*
* @see https://developer.wordpress.org/block-editor/reference-guides/slotfills/plugin-sidebar/
*/
import { registerPlugin } from '@wordpress/plugins';
import MetaBox from './parts/MetaBox';
This code is pretty self-explanatory. Nonetheless, we need to take a second to dwell on the 2 import
statements for these readers who would not have superior React expertise.
With the primary import
assertion, we enclosed the identify of the operate in curly brackets. With the second import
assertion, the identify of the part just isn’t enclosed in curly brackets.
Subsequent, register your plugin:
registerPlugin( 'metadata-plugin', {
render: MetaBox
} );
registerPlugin
merely registers a plugin. The operate accepts two parameters:
- A novel string that identifies the plugin
- An object of plugin settings. Observe that the
render
property have to be specified and have to be a sound operate.
Construct the Part Utilizing Constructed-in Gutenberg Elements
It’s time to construct our React part. Open the MetaBox.js file (or no matter you referred to as it) and add the next import statements:
import { __ } from '@wordpress/i18n';
import { compose } from '@wordpress/compose';
import { withSelect, withDispatch } from '@wordpress/knowledge';
import { PluginDocumentSettingPanel } from '@wordpress/edit-post';
import { PanelRow, TextControl, DateTimePicker } from '@wordpress/parts';
- The
compose
operate performs operate composition, that means that the results of a operate is handed on to a different operate.Earlier than you need to usecompose
, you could want to put in the corresponding module:npm set up @wordpress/compose --save
We’ll see the
compose
operate in motion in a second. withSelect
andwithDispatch
are two larger order parts that will let you fetch or dispatch knowledge from or to a WordPress retailer.withSelect
is used to inject state-derived props utilizing registered selectors,withDispatch
is used to dispatch props utilizing registered motion creators.PluginDocumentSettingPanel
renders objects within the Doc Sidebar (see the supply code on Github).
Subsequent, you’ll create the part to show the meta field panel within the Doc sidebar. In your MetaBox.js file, add the next code:
const MetaBox = ( { postType, metaFields, setMetaFields } ) => {
if ( 'submit' !== postType ) return null;
return(
<PluginDocumentSettingPanel
title={ __( 'Guide particulars' ) }
icon="e book"
initialOpen={ false }
>
<PanelRow>
<TextControl
worth={ metaFields._meta_fields_book_title }
label={ __( "Title" ) }
onChange={ (worth) => setMetaFields( { _meta_fields_book_title: worth } ) }
/>
</PanelRow>
<PanelRow>
<TextControl
worth={ metaFields._meta_fields_book_author }
label={ __( "Creator" ) }
onChange={ (worth) => setMetaFields( { _meta_fields_book_author: worth } ) }
/>
</PanelRow>
<PanelRow>
<TextControl
worth={ metaFields._meta_fields_book_publisher }
label={ __( "Writer" ) }
onChange={ (worth) => setMetaFields( { _meta_fields_book_publisher: worth } ) }
/>
</PanelRow>
<PanelRow>
<DateTimePicker
currentDate={ metaFields._meta_fields_book_date }
onChange={ ( newDate ) => setMetaFields( { _meta_fields_book_date: newDate } ) }
__nextRemoveHelpButton
__nextRemoveResetButton
/>
</PanelRow>
</PluginDocumentSettingPanel>
);
}
const applyWithSelect = withSelect( ( choose ) => {
return {
metaFields: choose( 'core/editor' ).getEditedPostAttribute( 'meta' ),
postType: choose( 'core/editor' ).getCurrentPostType()
};
} );
const applyWithDispatch = withDispatch( ( dispatch ) => {
return {
setMetaFields ( newValue ) {
dispatch('core/editor').editPost( { meta: newValue } )
}
}
} );
export default compose([
applyWithSelect,
applyWithDispatch
])(MetaBox);
Let’s break down this code.
- The
PluginDocumentSettingPanel
ingredient renders a brand new panel within the Doc sidebar. We set the title (“Guide particulars”) and icon, and setinitialOpen
tofalse
, which implies that initially the panel is closed. - Throughout the
PluginDocumentSettingPanel
we’ve three textual content fields and aDateTimePicker
ingredient that enables the consumer to set the publication date. withSelect
offers entry to thechoose
operate that we’re utilizing to retrievemetaFields
andpostType
.withDispatch
offers entry to thedispatch
operate, which permits to replace the metadata values.- Lastly, the
compose
operate permits us to compose our part withwithSelect
andwithDispatch
higher-order parts. This provides the part entry to themetaFields
andpostType
properties and thesetMetaFields
operate.
Save your MetaBox.js file and create a brand new submit in your WordPress growth web site and check out the Doc Sidebar. It’s best to see the brand new Guide particulars panel.
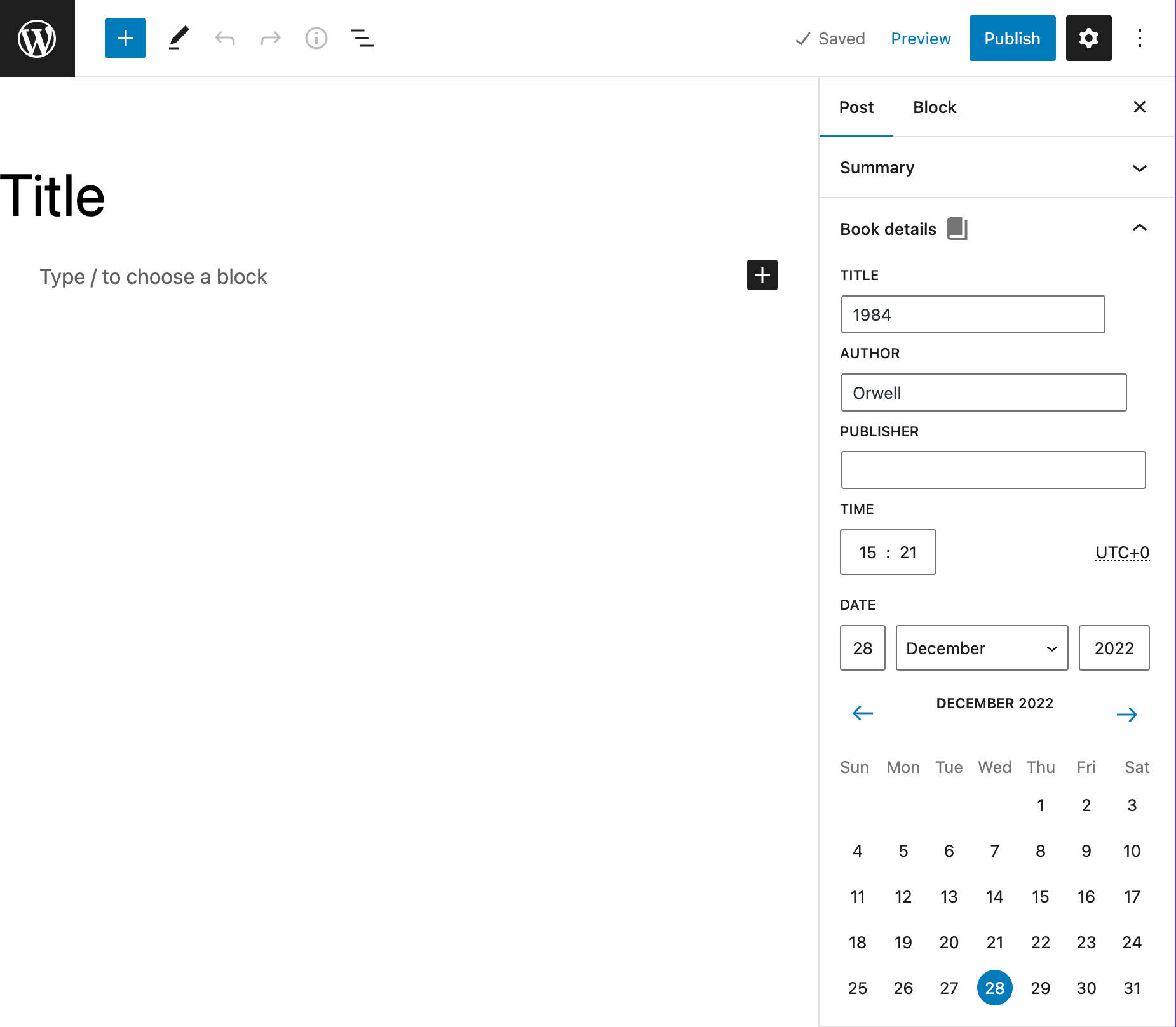
Now run your assessments. Set the values of your customized meta fields and save the submit. Then reload the web page and test if the values you entered are in place.
Add the block we’ve constructed within the earlier part and test if all the pieces is working correctly.
Including a Customized Sidebar To Handle Put up Meta Information
When you have numerous customized meta fields so as to add to your posts or customized submit sorts, you would additionally create a Customized Settings Sidebar particularly on your plugin.
The method is similar to the earlier instance, so when you’ve understood the steps mentioned within the earlier part, you received’t have any issue with constructing a Customized Sidebar for Gutenberg.
Once more:
- Create a brand new block plugin with create-block
- Register a customized meta field for the Basic Editor
- Register the customized meta fields in the primary plugin file through the register_post_meta() operate
- Register a plugin within the index.js file
- Construct the part utilizing built-in Gutenberg parts
Create a New Block Plugin With the create-block Device
Once more, to create a brand new block plugin, observe the steps mentioned above. You’ll be able to create a brand new plugin or edit the scripts constructed within the earlier examples.
Register a Customized Meta Field for the Basic Editor
Now register a customized meta field to make sure backward compatibility for WordPress web sites nonetheless utilizing the Basic Editor. The method is identical as described within the earlier part.
Register the Customized Meta Fields within the Most important Plugin File
Register the customized meta fields in the primary plugin file through the register_post_meta()
operate.
Register a Plugin within the index.js File
Now create an empty CustomSidebar.js file in your parts folder.
As soon as performed, change your index.js file as follows:
/**
* Registers a plugin for including objects to the Gutenberg Toolbar
*
* @see https://developer.wordpress.org/block-editor/reference-guides/slotfills/plugin-sidebar/
*/
import { registerPlugin } from '@wordpress/plugins';
import CustomSidebar from './parts/CustomSidebar';
// import MetaBox from './parts/MetaBox';
registerPlugin( 'metadata-block', {
render: CustomSidebar
} );
With the code above we first import the CustomSidebar
part, then we inform the registerPlugin
operate to render the brand new part.
Construct the Part Utilizing Constructed-in Gutenberg Elements
Subsequent, open the CustomSidebar.js file and add the next dependencies:
import { __ } from '@wordpress/i18n';
import { compose } from '@wordpress/compose';
import { withSelect, withDispatch } from '@wordpress/knowledge';
import { PluginSidebar, PluginSidebarMoreMenuItem } from '@wordpress/edit-post';
import { PanelBody, PanelRow, TextControl, DateTimePicker } from '@wordpress/parts';
It’s best to discover that we’re importing two new parts:
PluginSidebar
provides an icon into the Gutenberg Toolbar that, when clicked, shows a sidebar together with the content material wrapped within the<PluginSidebar />
ingredient (The part can be documented on GitHub).PluginSidebarMoreMenuItem
renders a menu merchandise underneath Plugins in Extra Menu dropdown and can be utilized to activate the correspondingPluginSidebar
part (see additionally on GitHub).
Now you possibly can construct your customized part:
const CustomSidebar = ( { postType, metaFields, setMetaFields } ) => {
if ( 'submit' !== postType ) return null;
return (
<>
<PluginSidebarMoreMenuItem
goal="metadata-sidebar"
icon="e book"
>
Metadata Sidebar
</PluginSidebarMoreMenuItem>
<PluginSidebar
identify="metadata-sidebar"
icon="e book"
title="My Sidebar"
>
<PanelBody title="Guide particulars" initialOpen={ true }>
<PanelRow>
<TextControl
worth={ metaFields._meta_fields_book_title }
label={ __( "Title" ) }
onChange={ (worth) => setMetaFields( { _meta_fields_book_title: worth } ) }
/>
</PanelRow>
<PanelRow>
<TextControl
worth={ metaFields._meta_fields_book_author }
label={ __("Creator", "textdomain") }
onChange={ (worth) => setMetaFields( { _meta_fields_book_author: worth } ) }
/>
</PanelRow>
<PanelRow>
<TextControl
worth={ metaFields._meta_fields_book_publisher }
label={ __("Writer", "textdomain") }
onChange={ (worth) => setMetaFields( { _meta_fields_book_publisher: worth } ) }
/>
</PanelRow>
<PanelRow>
<DateTimePicker
currentDate={ metaFields._meta_fields_book_date }
onChange={ ( newDate ) => setMetaFields( { _meta_fields_book_date: newDate } ) }
__nextRemoveHelpButton
__nextRemoveResetButton
/>
</PanelRow>
</PanelBody>
</PluginSidebar>
</>
)
}
The ultimate step is the part composition with withSelect
and withDispatch
higher-order parts:
const applyWithSelect = withSelect( ( choose ) => {
return {
metaFields: choose( 'core/editor' ).getEditedPostAttribute( 'meta' ),
postType: choose( 'core/editor' ).getCurrentPostType()
};
} );
const applyWithDispatch = withDispatch( ( dispatch ) => {
return {
setMetaFields ( newValue ) {
dispatch('core/editor').editPost( { meta: newValue } )
}
}
} );
export default compose([
applyWithSelect,
applyWithDispatch
])(CustomSidebar);
Save your modifications, then test the editor interface. If you happen to open the Choices dropdown, you’ll see a brand new Metadata Sidebar merchandise underneath the Plugins part. Clicking on the brand new merchandise will activate your brand-new customized sidebar.
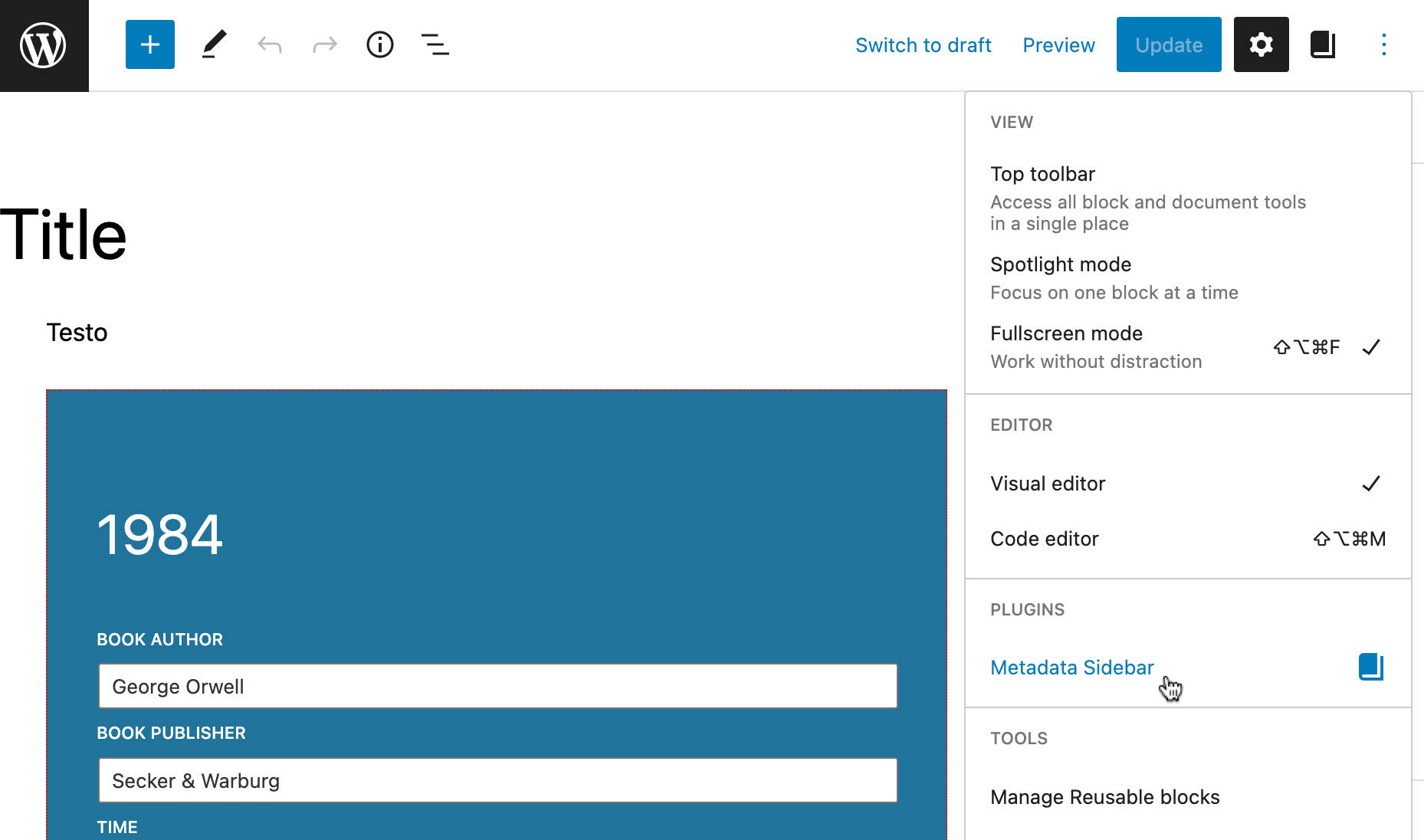
The identical occurs once you click on on the e book icon within the higher proper nook.
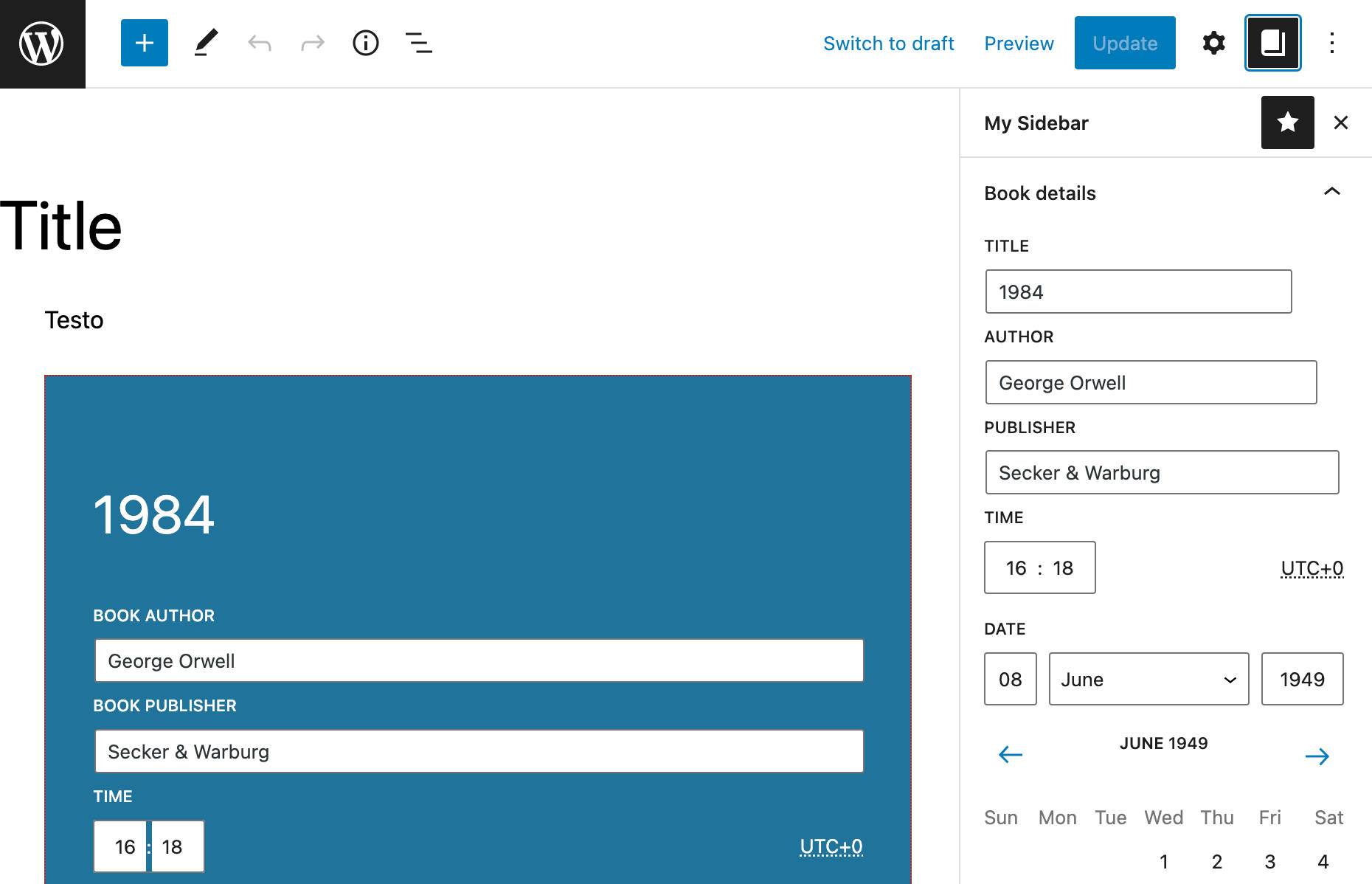
Now return to your growth web site, and create a brand new weblog submit. Fill in your meta fields, then add the block to the editor’s canvas. It ought to embody the identical meta values you entered in your customized sidebar.
Save the submit and preview the web page on the frontend. It’s best to see your card together with the e book title, creator, writer, and publication date.
You’ll discover the total code of this text on this public gist.
Additional Readings
On this article, we lined a number of subjects, from selectors to higher-order parts and way more. We’ve linked the highest sources we used for reference all through the article as effectively.
However when you want to dive deeper into these subjects, you may additionally need to test the next extra sources:
Gutenberg Documentation and Official WordPress Sources
Extra Official Sources
Further Sources From the Group
Helpful Readings From the Kinsta Web site
Abstract
On this third article in our sequence on Gutenberg block growth, we lined new superior subjects that ought to make the image outlined in earlier articles on static and dynamic block growth extra full.
It’s best to now be capable to benefit from the potential of customized fields in Gutenberg and create extra superior and purposeful WordPress web sites.
However there’s extra. With the abilities you’ve gained from our articles on block growth, you also needs to have a good suggestion of methods to develop React parts exterior of WordPress. In any case, Gutenberg is a React-based SPA.
And now it’s right down to you! Have you ever already created Gutenberg blocks that use customized meta fields? Share your creations with us within the feedback beneath.
Get all of your functions, databases and WordPress websites on-line and underneath one roof. Our feature-packed, high-performance cloud platform consists of:
- Straightforward setup and administration within the MyKinsta dashboard
- 24/7 knowledgeable help
- The very best Google Cloud Platform {hardware} and community, powered by Kubernetes for max scalability
- An enterprise-level Cloudflare integration for pace and safety
- International viewers attain with as much as 35 knowledge facilities and 275+ PoPs worldwide
Check it your self with $20 off your first month of Software Internet hosting or Database Internet hosting. Discover our plans or discuss to gross sales to seek out your greatest match.