Cease doing these errors in your Python code
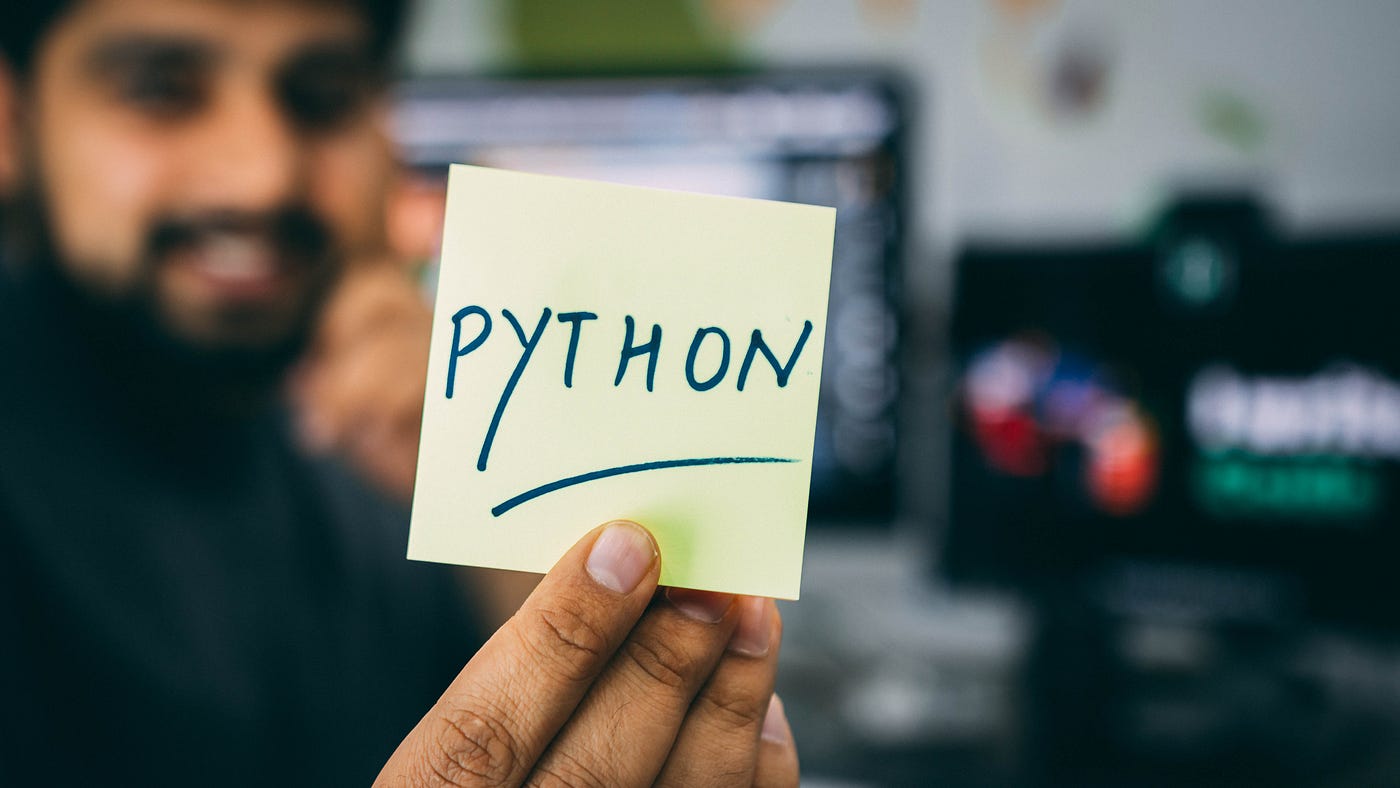
The journey of reaching good evaluation outcomes is stuffed with explorations, experimentations, and testing of a number of approaches. On this journey, we write plenty of codes that we and others discuss with whereas working as a crew. In case your code shouldn’t be clear sufficient, it’ll develop into a ache for others (and also you too) to grasp, reuse, or modify your code.
In the event you write a neat and clear code, you’ll thank your self and others will thanks too.
This weblog will introduce you to the Python coding finest practices for writing neat and clear code.
There are business requirements similar to PEP8 for python programming. PEP stands for Python Enhancement Proposal. Let’s take a look at these requirements intimately:
1. Variable Naming Conference
Setting intuitive variable names may be very useful for anybody to grasp ‘what’ we are attempting to do.
Usually, we create variables utilizing single characters similar to i, j, okay — these aren’t self-explanatory variables and must be averted.
Word: By no means use l, o, or I single-letter names as these could be mistaken for “1” and “0”, relying on the typeface.
o = 2 # This may occasionally appear like you are making an attempt to reassign 2 to zero
Dangerous Apply
x = 'Anmol Tomar'
y, z = x.break up()
print(z, y, sep=', ')
'Tomar, Anmol'
We will use underscore “_” to create multi phrases variable names as proven under:
Good Apply
identify = 'Anmol Tomar'
first_name, last_name = identify.break up()
print(last_name, first_name, sep=', ')
'Tomar, Anmol'
Word: Cease utilizing df to outline your information frames, as a substitute, use the extra intuitive names.
2. Feedback in operate
Feedback are one of the vital necessary facets of creating our code human-readable and simple to grasp.
There are 2 kinds of feedback:
- Inline Remark: Feedback that are in the identical line as our code. ‘#’ is used to set the inline feedback.
a = 10 # Single line remark
2. Block Remark: Multi-line feedback are known as block feedback.
""" Instance of Multi-line remark
Line 2 of a multiline remark
"""
Dangerous Apply
def function_name(param1, param2):
print(param1)
Good Apply
def function_name(param1, param2):
"""Instance operate with PEP 484 kind annotations.Args:
param1: The primary parameter.
param2: The second parameter.Returns:
The return worth. True for achievement, False in any other case."""
print(param1)
3. Indentation
Indentation is a strong characteristic to make the code simple to learn and comply with. Indentation is about utilizing 4 areas/tabs per indentation stage.
Some syntaxes require the code to have the indentation by default. For instance, the contents throughout the physique of the operate definition:
def operate(var_one):
print(var_one)
We will use the indentation for creating clear and easy-to-read multi-line operate constituents (similar to arguments, variables, and so on.).
Dangerous Apply
# Arguments in 2nd line aren't aligned with arguments within the 1st line.
func = function_name(var_one, var_two,
var_three, var_four)# Additional indentation required as indentation shouldn't be distinguishable - Arguments are having identical indentation as print operate
def function_name(
var_one, var_two, var_three,
var_four):
print(var_one)
Good Apply
# Arguments in 2nd line are aligned with arguments within the 1st line.
func = function_name(var_one, var_two,
var_three, var_four)# Add 4 areas (an additional stage of indentation) to differentiate arguments from the remaining.
def function_name(
var_one, var_two, var_three,
var_four):
print(var_one)
Tabs or Areas for indentation? Areas are the popular indentation methodology. Tabs must be used solely to stay per code that’s already indented with tabs. Python disallows mixing tabs and areas for indentation.
4. Most Line Size
As per the PEP8, the perfect apply is to restrict all strains to a most of 79 characters. 80–100 characters are additionally positive typically.
For the codes throughout the parentheses or brackets, Python assumes line continuation by default, whereas for different circumstances, we are able to use “”.
Dangerous Apply
#having size greater than 79 characters.
joined_df = pd.merge(countries_df, countries_lat_lon, on = 'CountryCode', how = 'internal')
Good Apply
#Having size lower than 79 characters - inside parenthesis line continuation is by default.
joined_df = pd.merge(countries_df, countries_lat_lon,
on = 'CountryCode', how = 'internal')# Utilizing for the road continuation
from mypackage import example1,
example2, example3
5. Imports on separate strains
It is strongly recommended to import new package deal on a separate line.
Dangerous Apply
import sys, os, pandas
Good Apply
import os
import sys
import pandas
6. Spacing Suggestions
Spacing additionally impacts the general consumer readability expertise. The next is the perfect apply round spacing :
At all times encompass the next binary operators with a single house on both facet: task (=
), augmented task (+=
, -=,
and so on.), comparisons (==
, <
, >
, !=
, <>
, <=
, >=
, in
, not in
, is
, shouldn't be
), Booleans (and
, or
, not
).
Dangerous Apply
i=i+1
submitted +=1
x = x * 2 - 1
hypot2 = x * x + y * y
c = (a + b) * (a - b)
Good Apply
i = i + 1
submitted += 1
x = x*2 - 1
hypot2 = x*x + y*y
c = (a+b) * (a-b)