Tailwind and React are two main applied sciences of their sphere. Tailwind CSS simplifies the idea of Atomic CSS, enabling builders to fashion their UI by including some lessons to their markup. And with the efficiency enhancements that include the brand new JIT compiler, Tailwind CSS has develop into a transparent developer’s favourite.
Vite additionally simplifies the bundling course of however takes a distinct strategy than conventional bundlers. Vite provides us immediate dev sever begin occasions and extremely quick bundling by leveraging native JavaScript modules and esbuild beneath the hood.
Each Vite and Tailwind CSS excel in simplicity, efficiency, and developer expertise.
As software program turns into extra subtle to fulfill the wants of finish customers, app dimension will increase linearly and results in a requirement for quicker improvement instruments and bundlers. Though Create React App works effectively, because the app dimension will increase, its efficiency declines. That is the place Vite is available in.
On this article, we’ll find out about Vite and methods to arrange a React and Tailwind CSS utility utilizing it.
Background info
Traditionally, JavaScript didn’t have an API for builders to jot down code in a modular manner. It is because JavaScript was initially designed for small browser scripts.
Over time, JavaScript has develop into wildly fashionable and is utilized in totally different facets of programming. Nonetheless, its essential downside has been its lack of modularity. This has led JavaScript builders to provide you with alternative ways of making modules, corresponding to:
The issue is that not all module specs work within the browser. For instance, CJS helps solely server-side module declaration. Moreover, constructing a contemporary net utility includes the usage of some libraries and packages that aren’t supported by the browser, like React, Vue, TypeScript, and so forth.
This downside, nonetheless, is solved by the idea of bundling. The method includes utilizing a bundler (intuitive, proper?) to mix all our app’s recordsdata and belongings right into a single package deal that works within the browser. This has led to the event of conventional bundlers corresponding to webpack, Parcel, and Rollup.
There are two essential points with these: sluggish chilly begin of the dev server and sluggish updates. Subsequent technology JavaScript construct instruments, like Vite, determine and clear up these points for us.
What’s Vite?
Vite is the French phrase for quick. It’s is a contemporary construct instrument for frontend net improvement that leverages ES modules, or ESM. Vite contains a leaner and quicker bundler that comes with a preconfigured dev server.
Vite vs. webpack and conventional bundlers
As an ESM bundler, Vite solves the issues of conventional bundlers talked about above. We’ll stroll by just a few key differentiators beneath.
Chilly beginning apps
Not like webpack, Vite begins the dev server instantly after we chilly begin our utility, as seen beneath:
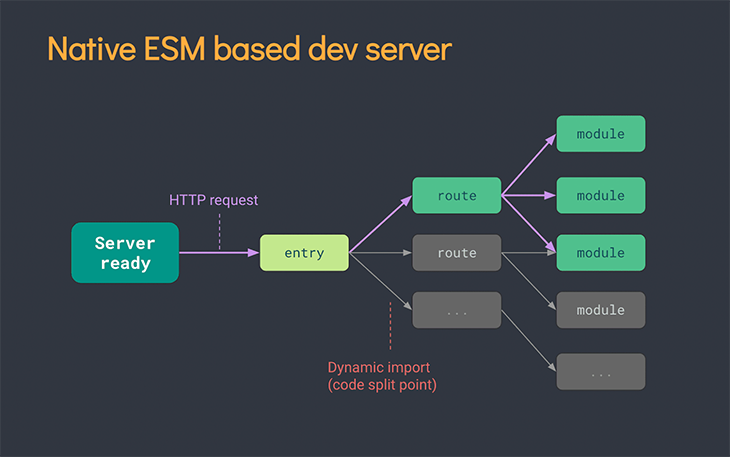
Vite can chilly begin the dev server immediately due to the next elements:
- Vite prebundles the app’s dependencies utilizing esbuild, constructed with Golang, making it 10–100x quicker than JavaScript bundlers. This redounds to the efficiency of Vite
- Vite dynamically determines which a part of the code must be loaded by utilizing route-based code splitting. Thus, Vite doesn’t should rebundle our whole utility
- Vite solely transforms and serves supply code requested by the browser. That is doable as a result of Vite serves our utility code over native ESM, enabling the browser to take over some a part of the bundling course of in improvement
Bundling course of
When in comparison with conventional bundlers like webpack, Vite takes a distinct strategy within the implementation particulars of its bundling course of. conventional bundlers like webpack rebuild the entire app on every replace. The primary downside with that is that it could actually get very costly.
To deal with this problem, these bundlers use a way referred to as Scorching Module Substitute, or HMR. HMR is a manner of updating the modified modules in a operating utility so that you don’t should replace the remainder of the web page.
Nonetheless, the replace pace of HMR decreases linearly because the app dimension grows.
Since Vite makes use of ESM, it performs HMR over ESM. This allows Vite to solely invalidate
the chain between the up to date module and its closest HMR boundary when a module is up to date. Thus, HMR in Vite is persistently quick whatever the dimension of the appliance.
Efficiency benefits
Vite makes use of the browser to hurry up full web page reloads by leveraging HTTP headers. It handles cache dependency module requests by way of Cache-Management: max-age=31536000, immutable
, so that they don’t hit the server once more.
Lastly, supply code module requests are made conditional by way of 304 Not Modified
.
All these give Vite a major efficiency benefit over bundle-based construct instruments.
Code splitting
One other main distinction between Vite and conventional bundlers is the dealing with of code splitting. Conventional bundlers like webpack and Rollup produce widespread chunk — code that’s shared between two or extra different chunks. This, when mixed with dynamic import, can result in a number of community spherical journeys as proven beneath:
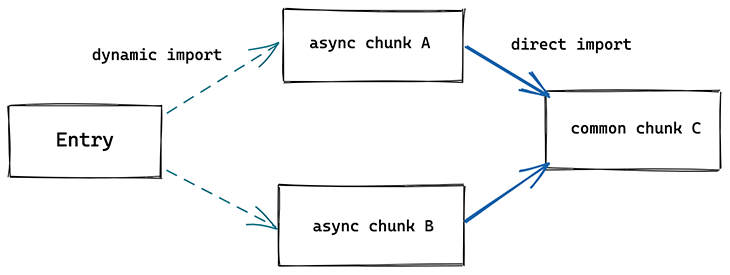
As proven within the picture, in unoptimized situations when async
chunk A
is imported, the browser has no manner of determining it wants widespread chunk C
with out first requesting and parsing A
. And after it figures out it wants widespread chunk C
, it then imports it, resulting in an additional community spherical journey.
Vite implements the code cut up dynamic import calls otherwise that provides a preload step. This manner, when chunk A
is requested, chunk C
is fetched in parallel. This fully eliminates community spherical journeys.
Compared with Create React App, Vite outshines it by way of efficiency for the explanations listed above. Though each are Node.js functions that may in the end obtain the identical factor, the one downside with Create React App is its efficiency.
Whereas Create React App works just for React, Vite is framework agnostic and helps lots of libraries and frameworks out of the field.
Within the subsequent part, we’ll learn to work with Vite by scaffolding a React utility with Vite.
Establishing a undertaking with React, Vite, and Tailwind
To scaffold a undertaking, run the next code out of your terminal:
npm create [email protected]
Select a undertaking identify and choose a template. Observe the on-screen directions to finish the setup, as seen beneath:
Alternatively, we are able to choose a template by utilizing the  — template
flag within the CLI
, as seen within the code beneath:
# npm 6.x npm create [email protected] my-vue-app --template vue # npm 7+, further double-dash is required: npm create [email protected] my-vue-app -- --template vue # npm 6.x npm create [email protected] my-react-app --template react # npm 7+, further double-dash is required: npm create [email protected] my-react-app -- --template react
Subsequent, set up the app dependencies and begin the dev server by operating:
# Installs dev dependencies npm set up #Begins dev server npm run dev
And we get:
Now we’ll combine Tailwind CSS. Probably the most seamless manner to do that is to make use of postCSS by following the steps beneath:
First, set up Tailwind CSS and its peer dependencies by operating:
npm set up -D tailwindcss postcss autoprefixer
Subsequent, create your tailwind.config.js
file by operating:
npx tailwindcss init
Add Tailwind to your postCSS configuration. To do that, create a postcss.config.js
file and add the next code:
module.exports = { plugins: { tailwindcss: {}, autoprefixer: {}, } }
Vite routinely applies all legitimate postCSS configuration within the postcss.config.js
object to all imported CSS.
Configure your template paths by modifying the tailwind.config.js
file as seen beneath:
module.exports = { content material:["./src/**/*.{js,jsx}"], theme: { prolong: {}, }, plugins: [], }
Add the Tailwind directives to your CSS by changing the code of your index.css
file with the next code:
@tailwind base; @tailwind elements; @tailwind utilities;
Now, run the construct course of by operating npm run dev
. You possibly can see that Tailwind CSS kinds have been utilized.
To see this in motion, we are able to apply some Tailwind CSS lessons to the counter app.
Within the src
listing, create a elements
listing.
Within the elements
listing, create a Counter.jsx
element with the next code:
import React, { useState } from "react"; const Counter = () => { const [count, setCount] = useState(0) return ( <div className="flex h-screen"> <div className="m-auto"> <div className="text-6xl text-red-600">{rely}</div> <button className="px-6 py-2 rounded bg-green-800 hover:bg-green-600 text-white" sort="button" onClick={() => setCount((rely) => rely + 1)}> rely+ </button> </div> </div> ) } export default Counter;
Now exchange the App.jsx
element with the next code:
import Counter from './elements/Counter' operate App() { return ( <div> <Counter /> </div> ) } export default App;
Now, we get:
And this confirms that we’ve efficiently bootstrapped our utility with Vite, React, and Tailwind CSS!
Conclusion
On this article, we discovered about Vite and the way it works. We in contrast Vite with conventional bundlers like webpack and noticed that Vite has some clear efficiency benefits, in addition to discovered methods to work with Vite by bootstrapping a React and Tailwind utility.
By following this, I do hope you might be prepared to provide Vite a strive in your subsequent React utility.
Full visibility into manufacturing React apps
Debugging React functions might be troublesome, particularly when customers expertise points which can be arduous to breed. In case you’re desirous about monitoring and monitoring Redux state, routinely surfacing JavaScript errors, and monitoring sluggish community requests and element load time, strive LogRocket.
LogRocket is sort of a DVR for net and cell apps, recording actually all the pieces that occurs in your React app. As an alternative of guessing why issues occur, you possibly can combination and report on what state your utility was in when a problem occurred. LogRocket additionally screens your app’s efficiency, reporting with metrics like consumer CPU load, consumer reminiscence utilization, and extra.
The LogRocket Redux middleware package deal provides an additional layer of visibility into your person classes. LogRocket logs all actions and state out of your Redux shops.
Modernize the way you debug your React apps — begin monitoring without cost.