Select your lists correctly
Everyone knows lists as a set of things, however there exist various list-like information buildings that may optimize your Python code relying on how you propose to make use of lists.
The default Python record does have its appeal — it’s easy, straightforward to grasp, and use. Nonetheless, after spending a while on LeetCode, I notice it might not be the greatest information construction in sure contexts. For instance on this LeetCode problem, holding the identical logic however merely utilizing one other information construction decreased my submission runtime from 99ms to 33ms! This may occasionally not appear to be so much, however think about the time saved if it had been to be scaled up. I need to additionally add that runtimes could be random and it might be incorrect to unexpectedly conclude that it all the time gives a 3x speedup.
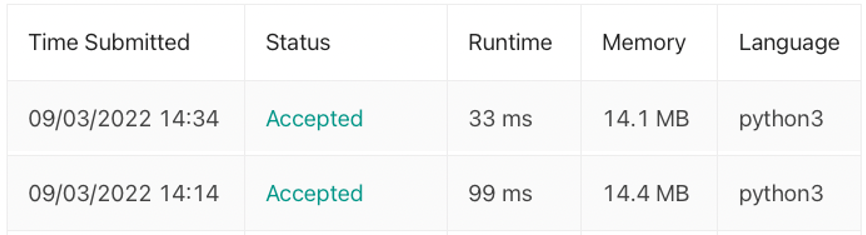
This text will introduce various list-like information buildings, when to make use of them, and easy methods to apply them with Python examples.
Use
heapq
for quicker retrieval of the smallest and largest objects in lists (and extra)
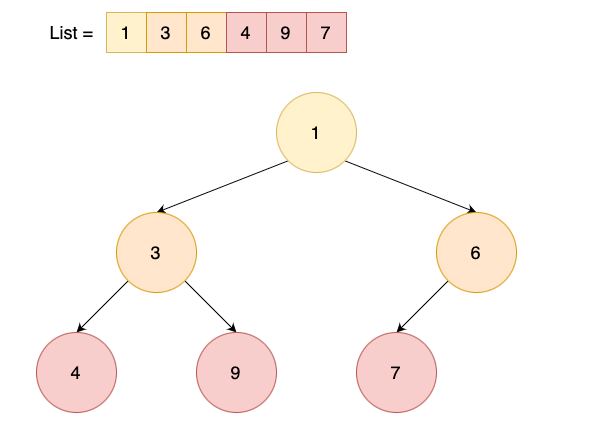
heapq
makes use of a heap queue algorithm the place a binary tree is designed such that the worth of any baby node is bigger than that of the guardian node. This leads to the smallest aspect being on the tree’s root node, and any insertion of a brand new merchandise will simply discover its place by ‘shuffling’ down the tree. After understanding how the binary tree is designed, it is very important observe that this doesn’t assure an precise sorted record, however merely a sorted binary tree. Heap queue is also called precedence queue as precedence is given to the merchandise on the prime of the tree, and eradicating that merchandise simply ‘shuffles’ or reprioritizes the remaining nodes.
To make a high-level comparability, in regular Python lists, objects are added in sequence such that appends
add a brand new merchandise to the again of the record and pop
removes and returns the final merchandise within the record. In heap queue construction, heappop
removes and returns the smallest merchandise within the record, whereas heappush
provides new objects to the record to take care of the sorted binary tree construction.
When to make use of heapq
,
- For retrieval and removing of the smallest merchandise in a listing
- For retrieval of n variety of the smallest and largest merchandise in a listing
- For preserving a sorted binary tree after including a brand new merchandise or merging two heaps
To make use of heapq
, we are able to rework an empty or populated record right into a heap,
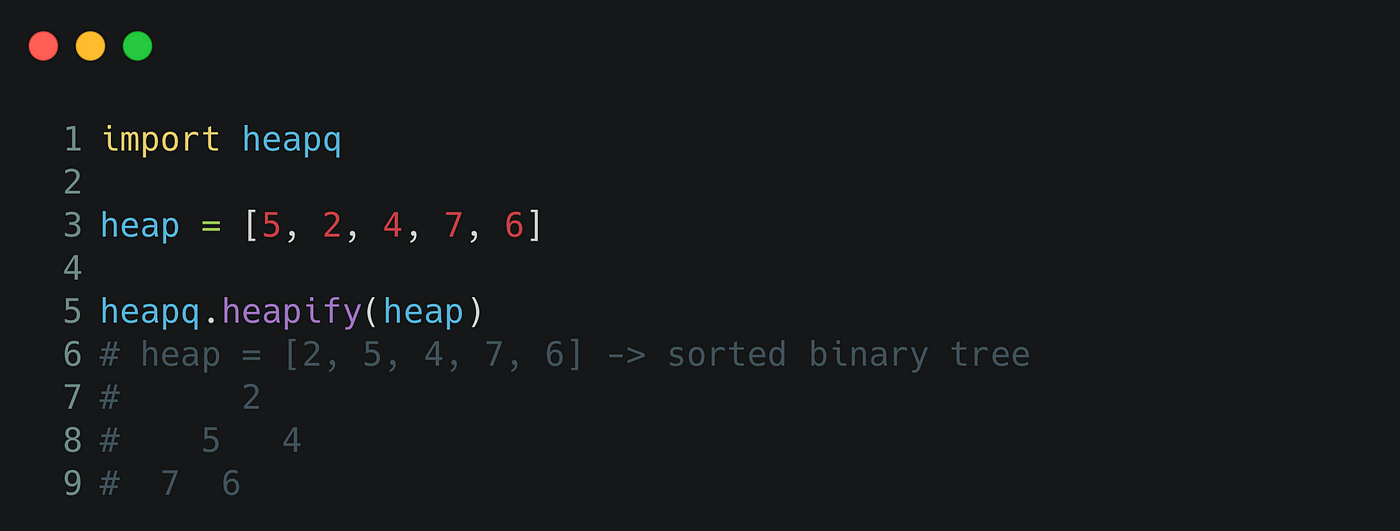
To remodel the heap, retrieval and addition of things to a heap could be achieved as such,

Lastly, to merge a number of heaps and protect the sorted binary tree construction, there’s a merge
technique obtainable. Do observe that the enter of merge
needs to be a heap (record following sorted binary tree construction) and never simply any record!

Use
bisect
for retrieving the closest worth in lists (and extra)
bisect
just isn’t a lot of an information construction, however a bisection algorithm that helps you find and/or insert objects right into a sorted record that maintains its order.
To offer a fast overview, Python makes use of a Timsort sorting algorithm which has a median of O(nlogn)
time-complexity. This sorting algorithm is quick, nevertheless, it might not be advisable to constantly name kind()
in your record, and is best to take care of a sorted record.
Do observe that the bisection algorithm solely works on an already-sorted record and wouldn’t carry out as anticipated on unsorted lists! Inserting an merchandise right into a (sorted) record could be achieved as follows,
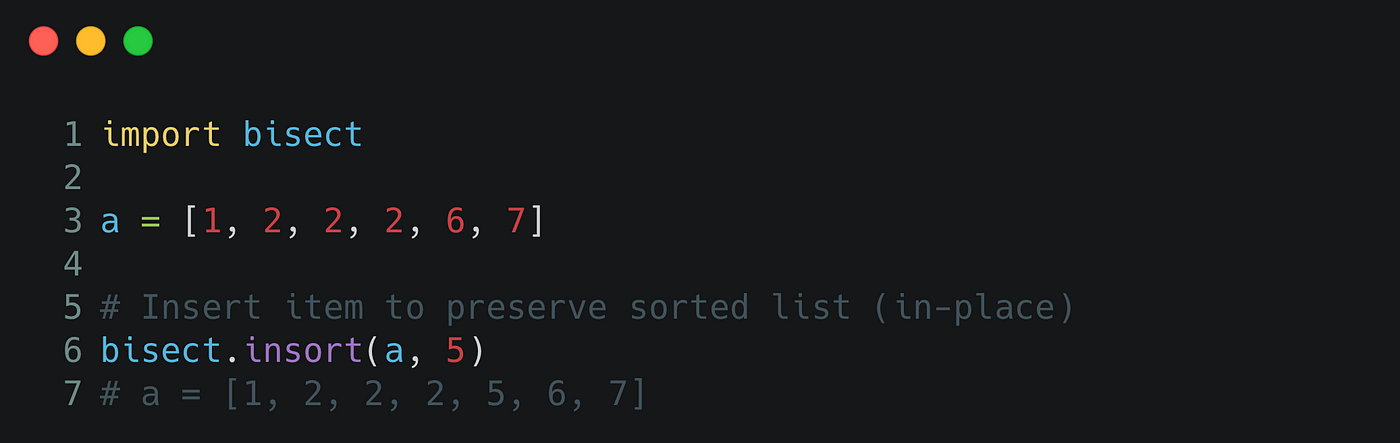
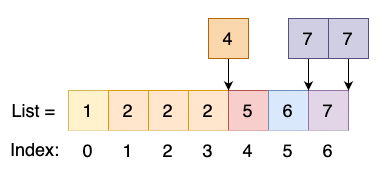
One other characteristic that I discover helpful in bisect
is that it may return the record index the place an merchandise needs to be inserted. In Determine 7, if 4
must be inserted into the sorted record, the bisect.bisect(record, 4)
technique will return index 4 the place it needs to be inserted. If the worth already exists, we are able to use bisect.bisect_left(record, 7)
or bisect.bisect_right(record, 7)
to point whether or not the brand new 7
needs to be inserted to the left or proper of the present worth(s) and return the index accordingly. By default, bisect()
implements bisect_right()
.
Retrieving the index utilizing bisect()
can also be a fast solution to discover the merchandise in a listing that’s closest to the worth that you simply had been going to ‘insert’. For instance, if I need to discover the smallest quantity that’s bigger or equal to 4, I can use bisect_left()
to get the index and retrieve the worth accordingly.
When to make use of bisect
,
- For addition of things to a sorted record that preserves the order of the record
- For retrieval of the index to insert a price that preserves the order of the record
- For retrieval of the smallest worth that’s bigger than
x
in a sorted record - For retrieval of the most important worth that’s smaller than
x
in a sorted record
Use
deque
for implementing a queue-like record
deque
stands for a doubly-ended queue and permits fast addition and removing of things at the back and front of a listing, making it straightforward to symbolize queueing buildings. It is usually attainable to implement a capability for the record to take away objects in entrance upon including objects on the again, and vice versa, when the record is at capability.
For comparability functions, deque
gives O(1)
time complexity for merchandise addition and removing in comparison with O(n)
time complexity in regular Python lists.
Regular record operations resembling index
, rely
, lengthen
, reverse
, take away,
and so on. nonetheless work in deque
.
When to make use of deque
,
- For addition of things to the entrance and/or again of a listing
- For removing of things from the entrance and/or again of a listing
- For shifting objects from the again of the queue to the entrance of the queue, and vice versa (round queue)
- For implementing record capability
Beneath are some examples of easy methods to work with deque
,
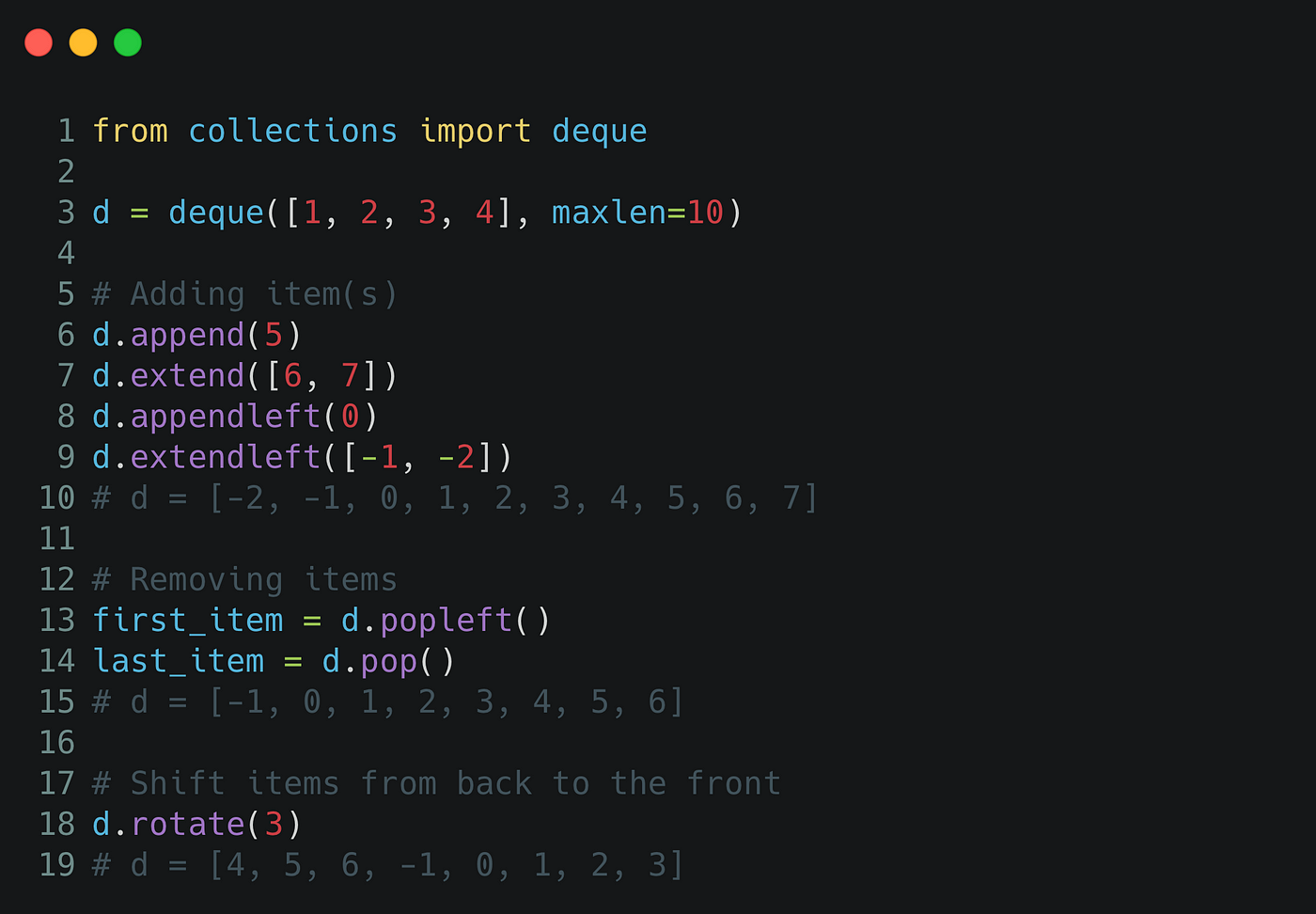
Hope you have got understood extra list-like information buildings and algorithms and may use them subsequent time to hurry up your code! It’s also possible to problem your self and attempt to implement these information buildings by hand, resembling this LeetCode problem for designing a deque
information construction.